說明
使用 chrome.fileBrowserHandler
API 擴充 ChromeOS 檔案瀏覽器。舉例來說,您可以使用這個 API 讓使用者將檔案上傳至網站。
概念與用法
使用者按下 Alt + Shift + M 或連接外部儲存裝置 (例如 SD 卡、USB 隨身碟、外接硬碟或數位相機) 時,ChromeOS 檔案瀏覽器就會顯示。除了顯示外部裝置上的檔案,檔案瀏覽器還能顯示使用者先前儲存至系統的檔案。
當使用者選取一或多個檔案時,檔案瀏覽器會新增代表這些檔案有效處理常式的按鈕。舉例來說,在下列螢幕截圖中,選取副檔名為「.png」的檔案後,使用者就能看到「Save to Gallery」按鈕。
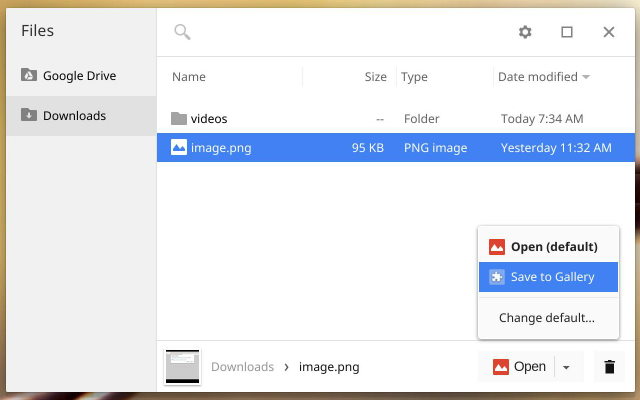
權限
fileBrowserHandler
您必須在擴充功能資訊清單中宣告 "fileBrowserHandler"
權限。
可用性
您必須使用 "file_browser_handlers"
欄位,將擴充功能註冊為至少一種檔案類型的處理常式。您也應提供 16 x 16 圖示,以便顯示在按鈕上。例如:
{
"name": "My extension",
...
"file_browser_handlers": [
{
"id": "upload",
"default_title": "Save to Gallery", // What the button will display
"file_filters": [
"filesystem:*.jpg", // To match all files, use "filesystem:*.*"
"filesystem:*.jpeg",
"filesystem:*.png"
]
}
],
"permissions" : [
"fileBrowserHandler"
],
"icons": {
"16": "icon16.png",
"48": "icon48.png",
"128": "icon128.png"
},
...
}
實作檔案瀏覽器處理常式
如要使用這個 API,您必須實作函式,以便處理 chrome.fileBrowserHandler
的 onExecute
事件。使用者點選代表檔案瀏覽器處理常式的按鈕時,系統就會呼叫您的函式。在函式中使用 File System API 取得檔案內容存取權。範例如下:
chrome.fileBrowserHandler.onExecute.addListener(async (id, details) => {
if (id !== 'upload') {
return; // check if you have multiple file_browser_handlers
}
for (const entry of detail.entries) {
// the FileSystemFileEntry doesn't have a Promise API, wrap in one
const file = await new Promise((resolve, reject) => {
entry.file(resolve, reject);
});
const buffer = await file.arrayBuffer();
// do something with buffer
}
});
事件處理常式會傳遞兩個引數:
id
- 資訊清單檔案中的
id
值。如果擴充功能實作多個處理常式,您可以查看 ID 值,瞭解系統觸發了哪個處理常式。 details
- 描述事件的物件。您可以從這個物件的
entries
欄位取得使用者選取的檔案,該欄位是FileSystemFileEntry
物件的陣列。
類型
FileHandlerExecuteEventDetails
fileBrowserHandler.onExecute 事件的事件詳細資料酬載。
屬性
-
項目
any[]
代表此動作目標檔案的項目例項陣列 (在 ChromeOS 檔案瀏覽器中選取)。
-
tab_id
號碼 選填
觸發此事件的分頁 ID。分頁 ID 在瀏覽器工作階段中是唯一的。
活動
onExecute
chrome.fileBrowserHandler.onExecute.addListener(
callback: function,
)
在 ChromeOS 檔案瀏覽器執行檔案系統動作時觸發。
參數
-
回呼
函式
callback
參數如下所示:(id: string, details: FileHandlerExecuteEventDetails) => void
-
id
字串
-