With the recent introduction of the Document Picture-in-Picture API (and even before), web developers are increasingly interested in being able to automatically open a picture-in-picture window when the user switches focus from their current tab. This is especially useful for video conferencing web apps, where it allows presenters to see and interact with participants in real time while presenting a document or using other tabs or windows.
Enter picture-in-picture automatically
To support these video conferencing use cases, from Chrome 120 desktop web apps can automatically enter picture-in-picture, with a few restrictions to ensure a positive user experience. A web app is only eligible for automatic picture-in-picture if it meets all of the following conditions:
It has registered a media session action handler for the
"enterpictureinpicture"
action.It is actively capturing camera or microphone via getUserMedia.
The user allows "automatic picture-in-picture" through a browser setting enabled by default.
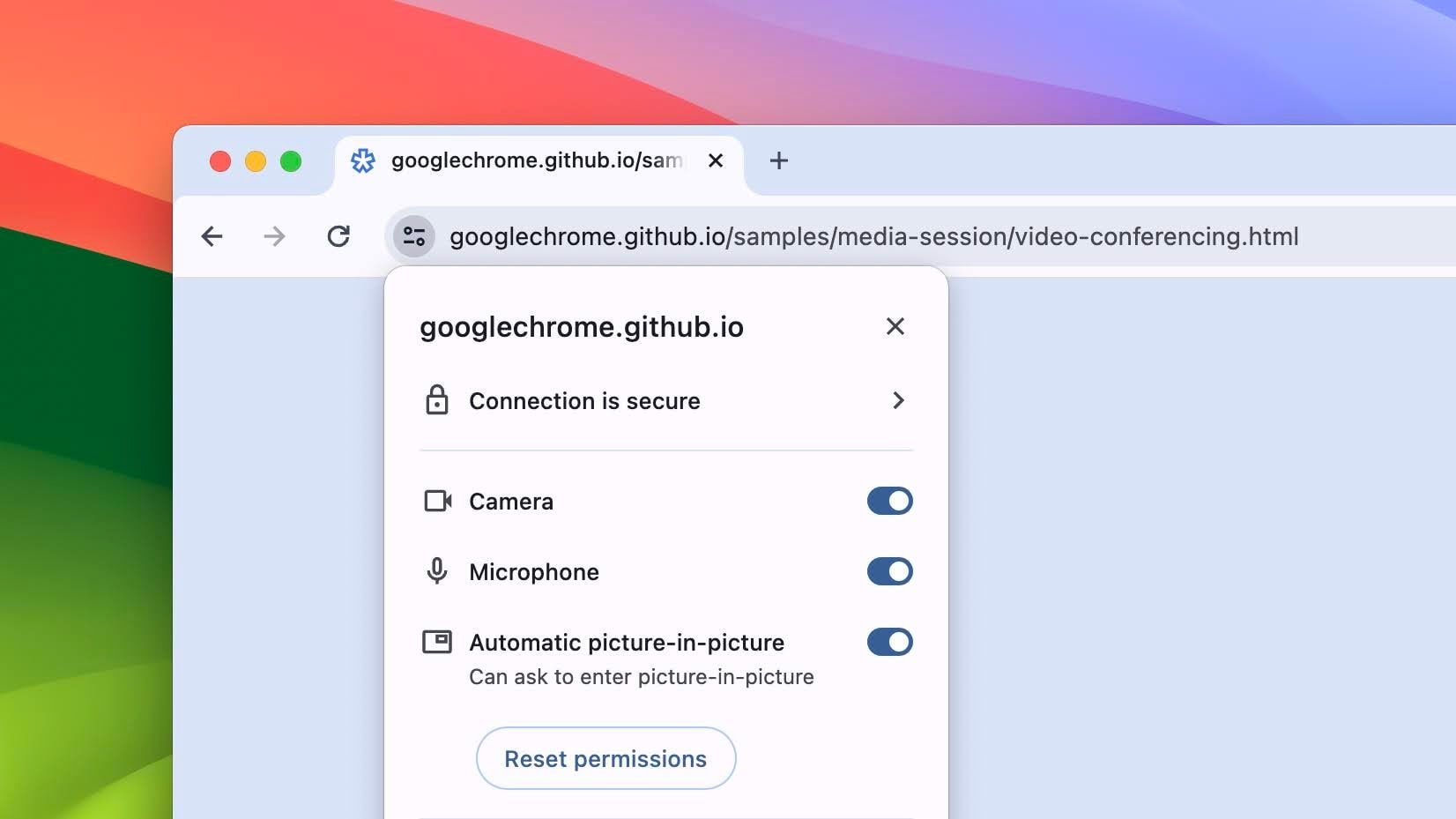
When a web app is eligible, the media session action handler callback function for the "enterpictureinpicture"
action is fired when the user switches focus to another tab, allowing it to open a picture-in-picture window without a user gesture.
Web developers can either use the Picture-in-Picture API for <video> to open a picture-in-picture window from an HTML <video> element, or the Document Picture-in-Picture API to open an always-on-top window to populate with arbitrary HTML content. The picture-in-picture window is not focused when opened and automatically closed when the page visibility becomes visible again.
The following example shows you how to request access to the user’s camera. Then, safely register a media session action handler for the "enterpictureinpicture"
action with a callback function that opens a picture-in-picture window. This window contains the user’s camera video stream with the Picture-in-Picture API for <video>.
const video = document.querySelector("video");
// Request access to the user's camera.
navigator.mediaDevices.getUserMedia({ video: true }).then((stream) => {
video.srcObject = stream;
});
try {
// Request video to automatically enter picture-in-picture when eligible.
navigator.mediaSession.setActionHandler("enterpictureinpicture", () => {
video.requestPictureInPicture();
});
} catch (error) {
console.log("The enterpictureinpicture action is not yet supported.");
}
Try the Video Conferencing Media Session sample.
Enter picture-in-picture from browser media control
The "enterpictureinpicture"
media session action is also useful when the user wants to enter picture-in-picture using the media control in the Chrome browser UI.
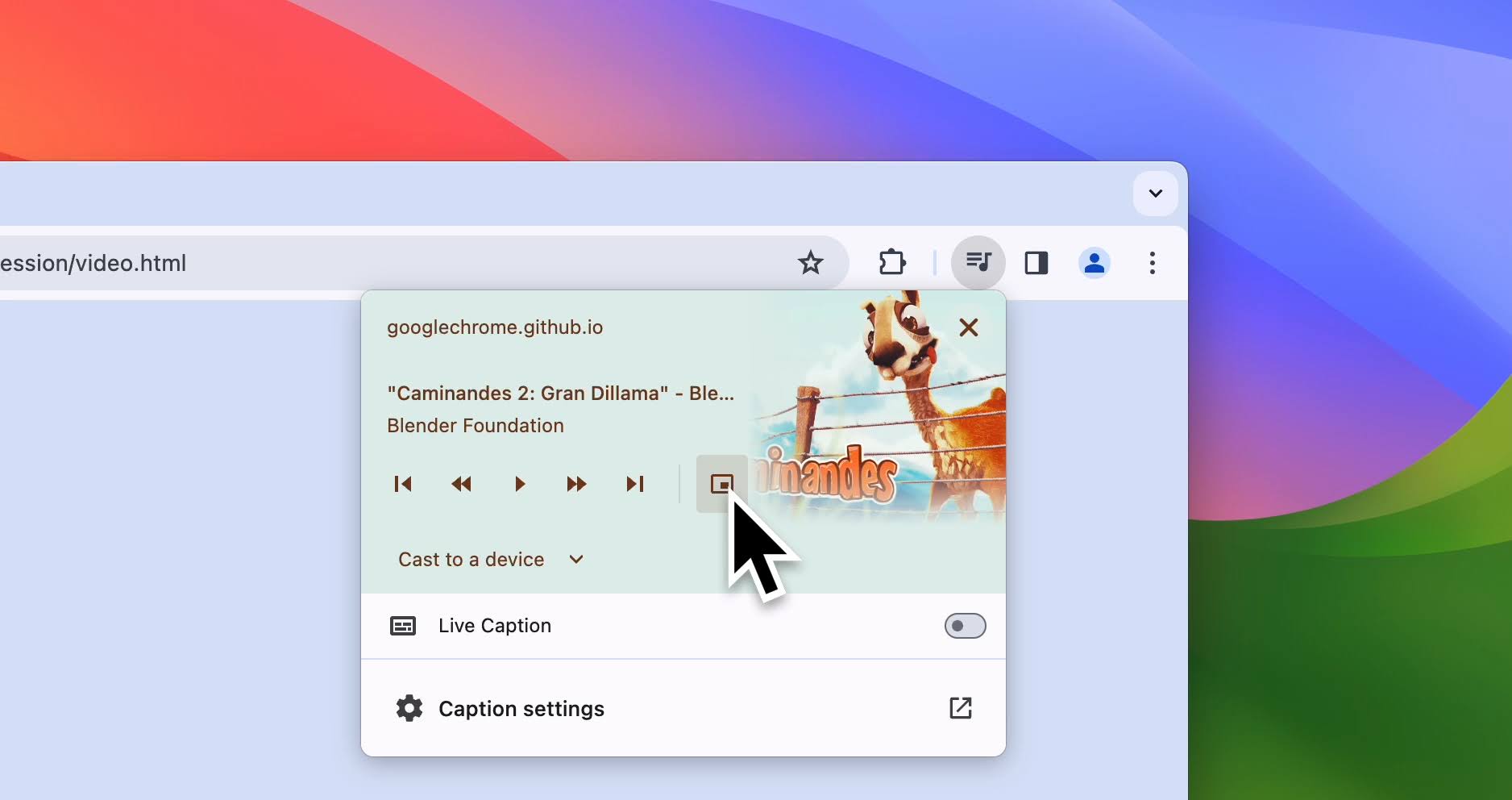
When there is no HTML <video> element playing but only audio, registering the media session action handler for "enterpictureinpicture"
tells the browser that the web app knows how to handle it and will take care of opening a picture-in-picture window itself.
It is also useful when the web app wants to use the Document Picture-in-Picture API to open a picture-in-picture window instead of letting the browser handling it with the Picture-in-Picture API for <video>.
The following example demonstrates how to safely register a media session action handler for the "enterpictureinpicture"
action. The callback function opens a picture-in-picture window with the Document Picture-in-Picture API when the user enters picture-in-picture using the media control in the Chrome browser UI.
try {
// Use the Document Picture-in-Picture API when entering
// picture-in-picture from browser media control.
navigator.mediaSession.setActionHandler("enterpictureinpicture", async () => {
const pipWindow = await documentPictureInPicture.requestWindow();
// Populate HTML content and handle closing window...
});
} catch (error) {
console.log("The enterpictureinpicture action is not yet supported.");
}
Try the Document Picture-in-Picture API VideoJS player demo or the Video Media Session sample.
Engage and share feedback
If you have feedback or encounter any issues, you can share them at crbug.com.
Resources
Acknowledgments
Thanks to Tommy Steimel, Ryan Flores, Shimi Rahim, Frank Liberato, and Rachel Andrew for their reviews.