はじめに
メディア ソース拡張機能(MSE)は、HTML5 の <audio>
要素と <video>
要素のバッファリングと再生を拡張します。元々は Dynamic Adaptive Streaming over HTTP(DASH) ベースの動画プレーヤーを容易にするために開発されましたが、以下では、音声、特にギャップレス再生にどのように使用できるかについて説明します。
曲がシームレスにつながる音楽アルバムを聴いたことがあるでしょう。今まさに聴いているかもしれません。アーティストは、芸術的な選択として、また、音声が 1 つの連続したストリームとして書き込まれたレコードやCD のアーティファクトとして、これらのギャップレス再生を作成します。残念ながら、MP3 や AAC などの最新のオーディオ コーデックの動作方法により、このシームレスな音声体験は失われることがよくあります。
理由については後で詳しく説明しますが、まずはデモから始めましょう。以下は、優れた Sintel の最初の 30 秒を 5 つの MP3 ファイルに分割し、MSE を使用して再構成したものです。赤い線は、各 MP3 の作成(エンコード)中に発生したギャップを示しています。これらのポイントでグリッチが発生します。
うわあ!ご不便をおかけして申し訳ございません。少し手間をかければ、上記のデモで使用した MP3 ファイルとまったく同じファイルを使用して、MSE で不要なギャップを削除できます。次のデモの緑色の線は、ファイルが結合され、ギャップが削除された場所を示しています。Chrome 38 以降では、シームレスに再生されます。
ギャップレス コンテンツを作成する方法はさまざまです。このデモでは、一般的なユーザーが保有している可能性のあるファイルの種類に焦点を当てます。各ファイルが、前後の音声セグメントに関係なく個別にエンコードされている場合。
基本的な設定
まず、MediaSource
インスタンスの基本的な設定について説明します。メディアソース拡張機能は、その名の通り、既存のメディア要素を拡張するものです。以下では、標準の URL を設定するときと同様に、MediaSource
インスタンスを表す Object URL
を音声要素の source 属性に割り当てています。
var audio = document.createElement('audio');
var mediaSource = new MediaSource();
var SEGMENTS = 5;
mediaSource.addEventListener('sourceopen', function() {
var sourceBuffer = mediaSource.addSourceBuffer('audio/mpeg');
function onAudioLoaded(data, index) {
// Append the ArrayBuffer data into our new SourceBuffer.
sourceBuffer.appendBuffer(data);
}
// Retrieve an audio segment via XHR. For simplicity, we're retrieving the
// entire segment at once, but we could also retrieve it in chunks and append
// each chunk separately. MSE will take care of assembling the pieces.
GET('sintel/sintel_0.mp3', function(data) { onAudioLoaded(data, 0); } );
});
audio.src = URL.createObjectURL(mediaSource);
MediaSource
オブジェクトが接続されると、いくつかの初期化が行われ、最終的に sourceopen
イベントがトリガーされます。この時点で SourceBuffer
を作成できます。上記の例では、MP3 セグメントを解析してデコードできる audio/mpeg
を作成しています。他にもいくつかのタイプがあります。
異常な波形
コードについては後で説明しますが、ここでは、追加したファイルの末尾を詳しく見てみましょう。以下は、sintel_0.mp3
トラックの両方のチャンネルで平均化された最後の 3, 000 サンプルのグラフです。赤い線の各ピクセルは、[-1.0, 1.0]
の範囲内の浮動小数点サンプルです。
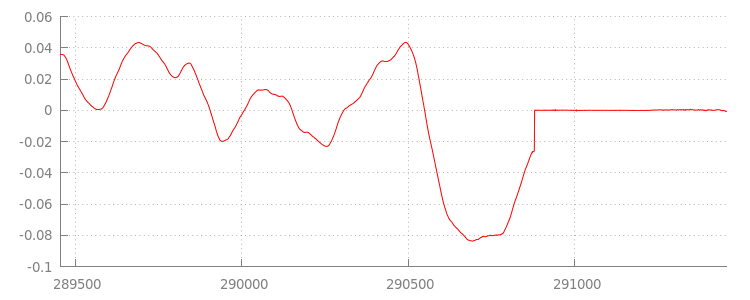
ゼロ(サイレント)のサンプルが大量に含まれているのはなぜですか?実際には、エンコード中に発生した圧縮アーティファクトが原因です。ほとんどのエンコーダはなんらかのタイプのパディングを導入しています。この場合、LAME はファイルの末尾に正確に 576 個のパディング サンプルを追加しました。
各ファイルの末尾に加えて、先頭にもパディングが追加されていました。sintel_1.mp3
トラックを先に確認すると、先頭に 576 サンプルのパディングがあることがわかります。パディングの量はエンコーダとコンテンツによって異なりますが、各ファイルに含まれる metadata
に基づいて正確な値を把握できます。
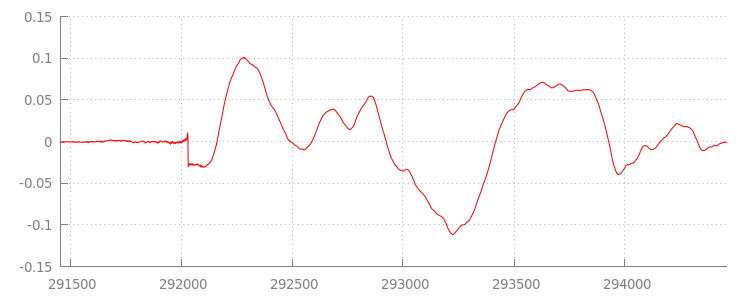
前のデモでセグメント間にグリッチが発生するのは、各ファイルの先頭と末尾にある無音のセクションが原因です。ギャップレス再生を実現するには、これらの無音部分を削除する必要があります。幸い、これは MediaSource
で簡単に行えます。以下では、アペンド ウィンドウとタイムスタンプ オフセットを使用してこの無音を削除するように onAudioLoaded()
メソッドを変更します。
コード例
function onAudioLoaded(data, index) {
// Parsing gapless metadata is unfortunately non trivial and a bit messy, so
// we'll glaze over it here; see the appendix for details.
// ParseGaplessData() will return a dictionary with two elements:
//
// audioDuration: Duration in seconds of all non-padding audio.
// frontPaddingDuration: Duration in seconds of the front padding.
//
var gaplessMetadata = ParseGaplessData(data);
// Each appended segment must be appended relative to the next. To avoid any
// overlaps, we'll use the end timestamp of the last append as the starting
// point for our next append or zero if we haven't appended anything yet.
var appendTime = index > 0 ? sourceBuffer.buffered.end(0) : 0;
// Simply put, an append window allows you to trim off audio (or video) frames
// which fall outside of a specified time range. Here, we'll use the end of
// our last append as the start of our append window and the end of the real
// audio data for this segment as the end of our append window.
sourceBuffer.appendWindowStart = appendTime;
sourceBuffer.appendWindowEnd = appendTime + gaplessMetadata.audioDuration;
// The timestampOffset field essentially tells MediaSource where in the media
// timeline the data given to appendBuffer() should be placed. I.e., if the
// timestampOffset is 1 second, the appended data will start 1 second into
// playback.
//
// MediaSource requires that the media timeline starts from time zero, so we
// need to ensure that the data left after filtering by the append window
// starts at time zero. We'll do this by shifting all of the padding we want
// to discard before our append time (and thus, before our append window).
sourceBuffer.timestampOffset =
appendTime - gaplessMetadata.frontPaddingDuration;
// When appendBuffer() completes, it will fire an updateend event signaling
// that it's okay to append another segment of media. Here, we'll chain the
// append for the next segment to the completion of our current append.
if (index == 0) {
sourceBuffer.addEventListener('updateend', function() {
if (++index < SEGMENTS) {
GET('sintel/sintel_' + index + '.mp3',
function(data) { onAudioLoaded(data, index); });
} else {
// We've loaded all available segments, so tell MediaSource there are no
// more buffers which will be appended.
mediaSource.endOfStream();
URL.revokeObjectURL(audio.src);
}
});
}
// appendBuffer() will now use the timestamp offset and append window settings
// to filter and timestamp the data we're appending.
//
// Note: While this demo uses very little memory, more complex use cases need
// to be careful about memory usage or garbage collection may remove ranges of
// media in unexpected places.
sourceBuffer.appendBuffer(data);
}
シームレスな波形
アペンド ウィンドウを適用した後の波形をもう一度見て、新しいコードがどのような成果をもたらしたかを確認しましょう。以下に、sintel_0.mp3
の末尾の無音セクション(赤)と sintel_1.mp3
の冒頭の無音セクション(青)が削除され、セグメント間のシームレスな遷移が実現されている様子を示します。
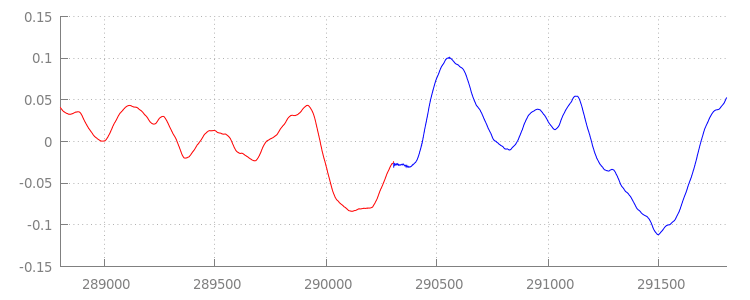
まとめ
これで、5 つのセグメントがすべてシームレスに 1 つに統合されました。デモはこれで終了です。最後に、onAudioLoaded()
メソッドではコンテナやコーデックが考慮されていないことに注意してください。つまり、これらの手法はすべて、コンテナやコーデックのタイプに関係なく機能します。以下では、MP3 ではなく、元のデモの DASH 対応の断片化された MP4 を再生できます。
詳しくは、以下の付録で、ギャップレス コンテンツの作成とメタデータの解析について詳しくご確認ください。gapless.js
で、このデモを動かすコードを詳しく確認することもできます。
今後ともよろしくお願いいたします。
付録 A: ギャップレス コンテンツを作成する
ギャップレス コンテンツの作成は難しい場合があります。以下では、このデモで使用する Sintel メディアの作成手順について説明します。まず、Sintel のロスレス FLAC サウンドトラックのコピーが必要です。後世のために、SHA1 を以下に示します。ツールには、FFmpeg、MP4Box、LAME、afconvert がインストールされた OSX が必要です。
unzip Jan_Morgenstern-Sintel-FLAC.zip
sha1sum 1-Snow_Fight.flac
# 0535ca207ccba70d538f7324916a3f1a3d550194 1-Snow_Fight.flac
まず、1-Snow_Fight.flac
トラックの最初の 31.5 秒を分割します。また、再生が終了した後にクリックが発生しないように、28 秒目から 2.5 秒間のフェードアウトを追加します。次の FFmpeg コマンドラインを使用すると、これらすべてを実行し、結果を sintel.flac
に格納できます。
ffmpeg -i 1-Snow_Fight.flac -t 31.5 -af "afade=t=out:st=28:d=2.5" sintel.flac
次に、ファイルを 6.5 秒の 5 つの wave ファイルに分割します。ほとんどのエンコーダが wave の取り込みをサポートしているため、wave を使用するのが最も簡単です。FFmpeg を使用すると、この処理を正確に行うことができます。処理が完了すると、sintel_0.wav
、sintel_1.wav
、sintel_2.wav
、sintel_3.wav
、sintel_4.wav
が作成されます。
ffmpeg -i sintel.flac -acodec pcm_f32le -map 0 -f segment \
-segment_list out.list -segment_time 6.5 sintel_%d.wav
次に、MP3 ファイルを作成します。LAME には、ギャップレス コンテンツを作成するオプションがいくつかあります。コンテンツを管理している場合は、すべてのファイルのバッチ エンコードで --nogap
を使用して、セグメント間のパディングを完全に回避することを検討してください。このデモでは、パディングが必要であるため、Wave ファイルの標準の高品質 VBR エンコードを使用します。
lame -V=2 sintel_0.wav sintel_0.mp3
lame -V=2 sintel_1.wav sintel_1.mp3
lame -V=2 sintel_2.wav sintel_2.mp3
lame -V=2 sintel_3.wav sintel_3.mp3
lame -V=2 sintel_4.wav sintel_4.mp3
これで、MP3 ファイルを作成するために必要な作業はすべて完了です。次に、断片化された MP4 ファイルの作成について説明します。iTunes 用にマスタリングされたメディアを作成するには、Apple の指示に沿って行います。以下では、手順に沿って Wave ファイルを中間 CAF ファイルに変換してから、推奨パラメータを使用して MP4 コンテナに AAC としてエンコードします。
afconvert sintel_0.wav sintel_0_intermediate.caf -d 0 -f caff \
--soundcheck-generate
afconvert sintel_1.wav sintel_1_intermediate.caf -d 0 -f caff \
--soundcheck-generate
afconvert sintel_2.wav sintel_2_intermediate.caf -d 0 -f caff \
--soundcheck-generate
afconvert sintel_3.wav sintel_3_intermediate.caf -d 0 -f caff \
--soundcheck-generate
afconvert sintel_4.wav sintel_4_intermediate.caf -d 0 -f caff \
--soundcheck-generate
afconvert sintel_0_intermediate.caf -d aac -f m4af -u pgcm 2 --soundcheck-read \
-b 256000 -q 127 -s 2 sintel_0.m4a
afconvert sintel_1_intermediate.caf -d aac -f m4af -u pgcm 2 --soundcheck-read \
-b 256000 -q 127 -s 2 sintel_1.m4a
afconvert sintel_2_intermediate.caf -d aac -f m4af -u pgcm 2 --soundcheck-read \
-b 256000 -q 127 -s 2 sintel_2.m4a
afconvert sintel_3_intermediate.caf -d aac -f m4af -u pgcm 2 --soundcheck-read \
-b 256000 -q 127 -s 2 sintel_3.m4a
afconvert sintel_4_intermediate.caf -d aac -f m4af -u pgcm 2 --soundcheck-read \
-b 256000 -q 127 -s 2 sintel_4.m4a
これで、MediaSource
で使用できるように、適切にフラグメント化する必要がある M4A ファイルがいくつかあります。ここでは、フラグメント サイズを 1 秒にします。MP4Box は、各断片化された MP4 を sintel_#_dashinit.mp4
として書き出し、破棄可能な MPEG-DASH マニフェスト(sintel_#_dash.mpd
)も書き出します。
MP4Box -dash 1000 sintel_0.m4a && mv sintel_0_dashinit.mp4 sintel_0.mp4
MP4Box -dash 1000 sintel_1.m4a && mv sintel_1_dashinit.mp4 sintel_1.mp4
MP4Box -dash 1000 sintel_2.m4a && mv sintel_2_dashinit.mp4 sintel_2.mp4
MP4Box -dash 1000 sintel_3.m4a && mv sintel_3_dashinit.mp4 sintel_3.mp4
MP4Box -dash 1000 sintel_4.m4a && mv sintel_4_dashinit.mp4 sintel_4.mp4
rm sintel_{0,1,2,3,4}_dash.mpd
これで、ギャップレス再生に必要な正しいメタデータが含まれた断片化された MP4 ファイルと MP3 ファイルが作成されます。メタデータの詳細については、付録 B をご覧ください。
付録 B: ギャップレス メタデータの解析
ギャップレス コンテンツの作成と同様に、ギャップレス メタデータの解析は、保存するための標準的な方法がないため、難しい場合があります。以下では、最も一般的な 2 つのエンコーダである LAME と iTunes がギャップレス メタデータを保存する方法について説明します。まず、いくつかのヘルパー メソッドを設定し、上記で使用した ParseGaplessData()
の概要を作成します。
// Since most MP3 encoders store the gapless metadata in binary, we'll need a
// method for turning bytes into integers. Note: This doesn't work for values
// larger than 2^30 since we'll overflow the signed integer type when shifting.
function ReadInt(buffer) {
var result = buffer.charCodeAt(0);
for (var i = 1; i < buffer.length; ++i) {
result <<../= 8;
result += buffer.charCodeAt(i);
}
return result;
}
function ParseGaplessData(arrayBuffer) {
// Gapless data is generally within the first 512 bytes, so limit parsing.
var byteStr = new TextDecoder().decode(arrayBuffer.slice(0, 512));
var frontPadding = 0, endPadding = 0, realSamples = 0;
// ... we'll fill this in as we go below.
解析と説明が最も簡単な Apple の iTunes メタデータ形式について、まず説明します。MP3 ファイルと M4A ファイル内では、iTunes(および afconvert)は次のように短いセクションを ASCII で書き込みます。
iTunSMPB[ 26 bytes ]0000000 00000840 000001C0 0000000000046E00
これは、MP3 コンテナ内の ID3 タグと、MP4 コンテナ内のメタデータ アトムに書き込まれます。ここでは、最初の 0000000
トークンは無視できます。次の 3 つのトークンは、前のパディング、後のパディング、パディング以外のサンプルの合計数です。これらの値を音声のサンプルレートで割ると、各フレームの時間がわかります。
// iTunes encodes the gapless data as hex strings like so:
//
// 'iTunSMPB[ 26 bytes ]0000000 00000840 000001C0 0000000000046E00'
// 'iTunSMPB[ 26 bytes ]####### frontpad endpad real samples'
//
// The approach here elides the complexity of actually parsing MP4 atoms. It
// may not work for all files without some tweaks.
var iTunesDataIndex = byteStr.indexOf('iTunSMPB');
if (iTunesDataIndex != -1) {
var frontPaddingIndex = iTunesDataIndex + 34;
frontPadding = parseInt(byteStr.substr(frontPaddingIndex, 8), 16);
var endPaddingIndex = frontPaddingIndex + 9;
endPadding = parseInt(byteStr.substr(endPaddingIndex, 8), 16);
var sampleCountIndex = endPaddingIndex + 9;
realSamples = parseInt(byteStr.substr(sampleCountIndex, 16), 16);
}
一方、ほとんどのオープンソース MP3 エンコーダでは、無音の MPEG フレーム内に配置された特別な Xing ヘッダー内に、ギャップレス メタデータを保存します(無音であるため、Xing ヘッダーを認識しないデコーダは単に無音を再生します)。残念ながら、このタグは常に存在するとは限らず、多くのオプション フィールドがあります。このデモではメディアを制御していますが、実際にギャップレス メタデータを使用できるタイミングを把握するには、実務上、追加のチェックが必要になります。
まず、サンプルの合計数を解析します。わかりやすくするため、ここでは Xing ヘッダーから読み取りますが、通常の MPEG オーディオ ヘッダーから構築することもできます。Xing ヘッダーは、Xing
タグまたは Info
タグでマークできます。このタグの 4 バイト後に、ファイル内のフレームの合計数を表す 32 ビットがあります。この値にフレームあたりのサンプル数を掛けると、ファイル内の合計サンプル数になります。
// Xing padding is encoded as 24bits within the header. Note: This code will
// only work for Layer3 Version 1 and Layer2 MP3 files with XING frame counts
// and gapless information. See the following document for more details:
// http://www.codeproject.com/Articles/8295/MPEG-Audio-Frame-Header
var xingDataIndex = byteStr.indexOf('Xing');
if (xingDataIndex == -1) xingDataIndex = byteStr.indexOf('Info');
if (xingDataIndex != -1) {
// See section 2.3.1 in the link above for the specifics on parsing the Xing
// frame count.
var frameCountIndex = xingDataIndex + 8;
var frameCount = ReadInt(byteStr.substr(frameCountIndex, 4));
// For Layer3 Version 1 and Layer2 there are 1152 samples per frame. See
// section 2.1.5 in the link above for more details.
var paddedSamples = frameCount * 1152;
// ... we'll cover this below.
サンプルの合計数がわかったので、次はパディング サンプルの数を読み取ります。エンコーダによっては、Xing ヘッダーにネストされた LAME タグまたは Lavf タグの下に書き込まれる場合があります。このヘッダーの 17 バイト後に、それぞれ 12 ビットの前後のパディングを表す 3 バイトがあります。
xingDataIndex = byteStr.indexOf('LAME');
if (xingDataIndex == -1) xingDataIndex = byteStr.indexOf('Lavf');
if (xingDataIndex != -1) {
// See http://gabriel.mp3-tech.org/mp3infotag.html#delays for details of
// how this information is encoded and parsed.
var gaplessDataIndex = xingDataIndex + 21;
var gaplessBits = ReadInt(byteStr.substr(gaplessDataIndex, 3));
// Upper 12 bits are the front padding, lower are the end padding.
frontPadding = gaplessBits >> 12;
endPadding = gaplessBits & 0xFFF;
}
realSamples = paddedSamples - (frontPadding + endPadding);
}
return {
audioDuration: realSamples * SECONDS_PER_SAMPLE,
frontPaddingDuration: frontPadding * SECONDS_PER_SAMPLE
};
}
これで、ギャップレス コンテンツのほとんどを解析する関数が完成しました。ただし、エッジケースは確かに多数存在するため、本番環境で同様のコードを利用する場合は注意が必要です。
付録 C: ガベージ コレクションについて
SourceBuffer
インスタンスに属するメモリは、コンテンツ タイプ、プラットフォーム固有の上限、現在の再生位置に応じてアクティブにガベージ コレクションされます。Chrome では、まずすでに再生されたバッファからメモリが再利用されます。ただし、メモリ使用量がプラットフォーム固有の上限を超えると、再生されていないバッファからメモリが削除されます。
再生がメモリの再利用によりタイムラインのギャップに達すると、ギャップが小さい場合はグリッチが発生し、ギャップが大きすぎる場合は再生が完全に停止することがあります。どちらもユーザー エクスペリエンスに悪影響を及ぼすため、一度に追加するデータが多すぎないようにし、不要になった範囲はメディア タイムラインから手動で削除することが重要です。
範囲は、各 SourceBuffer
の remove()
メソッドで削除できます。このメソッドは、秒単位の [start, end]
範囲を受け取ります。appendBuffer()
と同様に、各 remove()
は完了すると updateend
イベントを発生させます。その他の削除や追加は、イベントが発生するまで行わないでください。
デスクトップ版 Chrome では、一度に約 12 MB の音声コンテンツと 150 MB の動画コンテンツをメモリに保持できます。ブラウザやプラットフォーム間でこれらの値に依存しないでください。たとえば、モバイル デバイスを代表するものではありません。
ガベージ コレクションは、SourceBuffers
に追加されたデータにのみ影響します。JavaScript 変数にバッファに保持できるデータ量に上限はありません。必要に応じて、同じデータを同じ位置に再接続することもできます。