Chrome 66 では、ウェブページが Presentation API を介してセカンダリ接続ディスプレイを使用し、Presentation Receiver API を通じてそのコンテンツを制御できます。
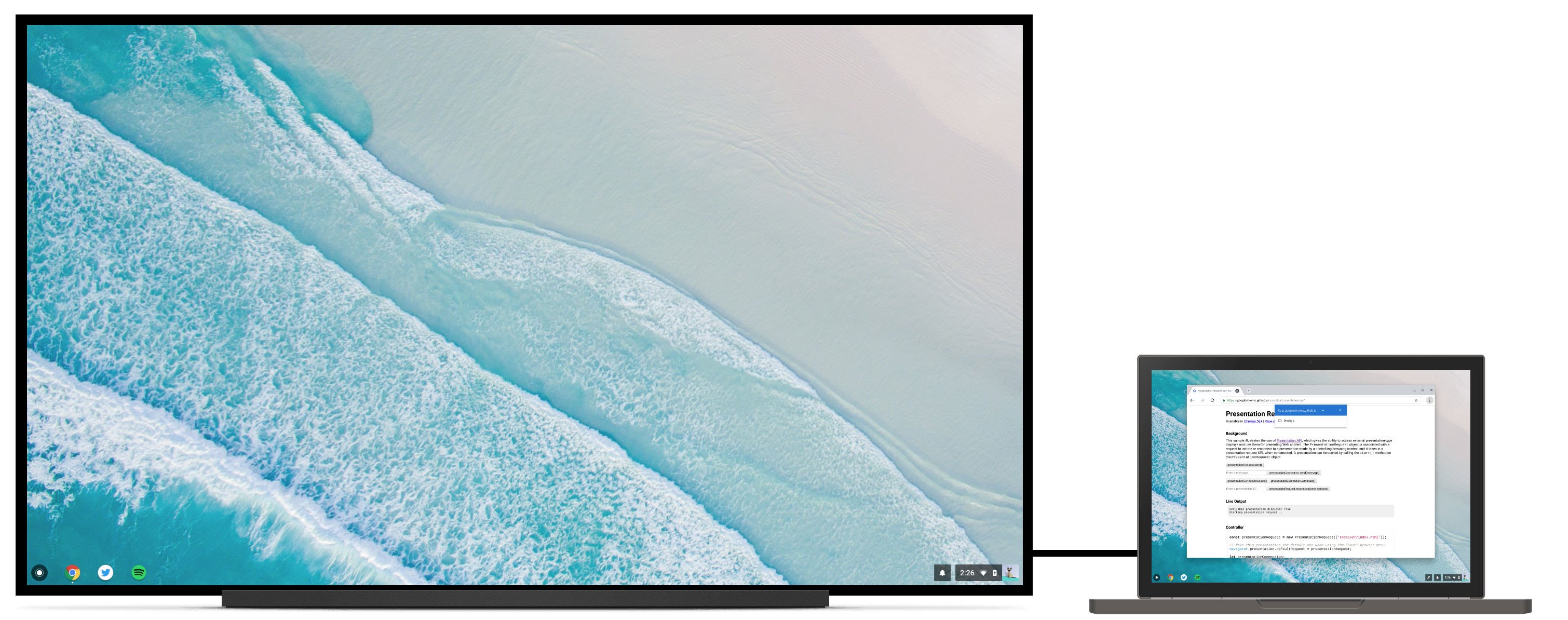
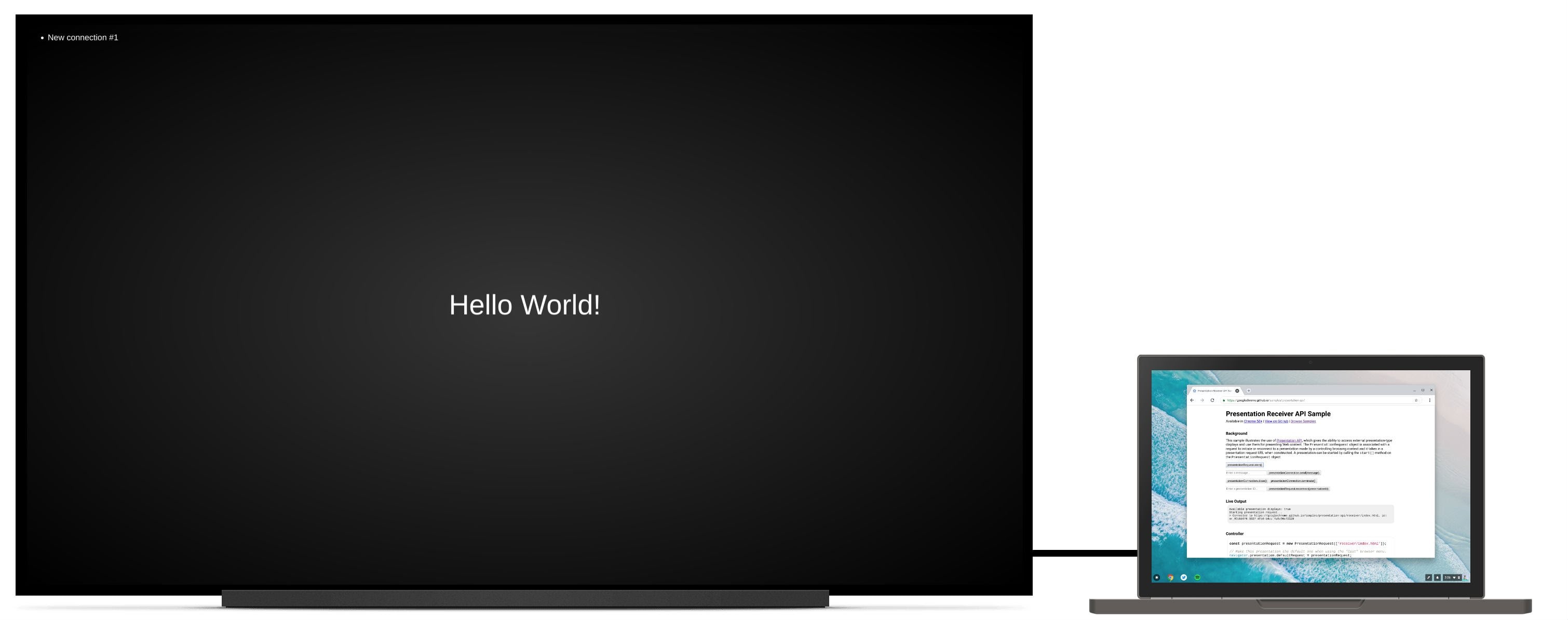
背景情報
これまでウェブ デベロッパーは、リモート ディスプレイに表示されるコンテンツとは異なるローカル コンテンツを Chrome でユーザーに表示され、そのコンテンツをローカルで制御できるエクスペリエンスを構築できました。たとえば、テレビで動画を再生しながら youtube.com の再生キューを管理したり、ノートパソコンでスピーカー ノート付きのスライドリールを表示したり、ハングアウト セッションで全画面プレゼンテーションを表示したりする場合などがこれに該当します。
ただし、単純に 2 台目のディスプレイにコンテンツを表示したいだけな場合もあります。たとえば、プロジェクターが設置された会議室にいるユーザーが HDMI ケーブルで接続しているとします。プレゼンテーションをリモート エンドポイントにミラーリングするのではなく、ユーザーはスライドをプロジェクターで全画面表示し、ノートパソコンの画面をスピーカー ノートやスライド操作に使用できるようにしたいと考えます。サイト作成者はこれをごく基本的な方法(たとえば、ユーザーが手動でセカンダリ ディスプレイにドラッグして全画面表示まで最大化する必要がある新しいウィンドウをポップアップする)でサポートできますが、面倒で、ローカルとリモートの表示間で一貫性がなくなります。
ページをプレゼンテーションする
Presentation API を使用して、セカンダリ接続ディスプレイにウェブページを表示する方法について説明します。最終結果は https://googlechrome.github.io/samples/presentation-api/ で確認できます。
まず、新しい PresentationRequest
オブジェクトを作成します。このオブジェクトには、セカンダリ接続ディスプレイに表示する URL を格納します。
const presentationRequest = new PresentationRequest('receiver.html');
In this article, I won’t cover use cases where the parameter passed to
`PresentationRequest` can be an array like `['cast://foo’, 'apple://foo',
'https://example.com']` as this is not relevant there.
We can now monitor presentation display availability and toggle a "Present"
button visibility based on presentation displays availability. Note that we can
also decide to always show this button.
<aside class="caution"><b>Caution:</b> The browser may use more energy while the <code>availability</code> object is alive
and actively listening for presentation display availability changes. Please
use it with caution in order to save energy on mobile.</aside>
```js
presentationRequest.getAvailability()
.then(availability => {
console.log('Available presentation displays: ' + availability.value);
availability.addEventListener('change', function() {
console.log('> Available presentation displays: ' + availability.value);
});
})
.catch(error => {
console.log('Presentation availability not supported, ' + error.name + ': ' +
error.message);
});
プレゼンテーションの表示プロンプトを表示するには、ボタンのクリックなどのユーザー操作が必要です。ボタンのクリックで presentationRequest.start()
を呼び出し、ユーザーがプレゼンテーション ディスプレイ(このユースケースではセカンダリ接続ディスプレイなど)を選択したら、Promise が解決されるのを待ちます。
function onPresentButtonClick() {
presentationRequest.start()
.then(connection => {
console.log('Connected to ' + connection.url + ', id: ' + connection.id);
})
.catch(error => {
console.log(error);
});
}
ユーザーに表示されるリストには、Chromecast デバイスなどのリモート エンドポイントも含めることができます(これらのエンドポイントをアドバタイズするネットワークに接続している場合)。ミラーリングされたディスプレイはリストに表示されません。http://crbug.com/840466 をご覧ください。
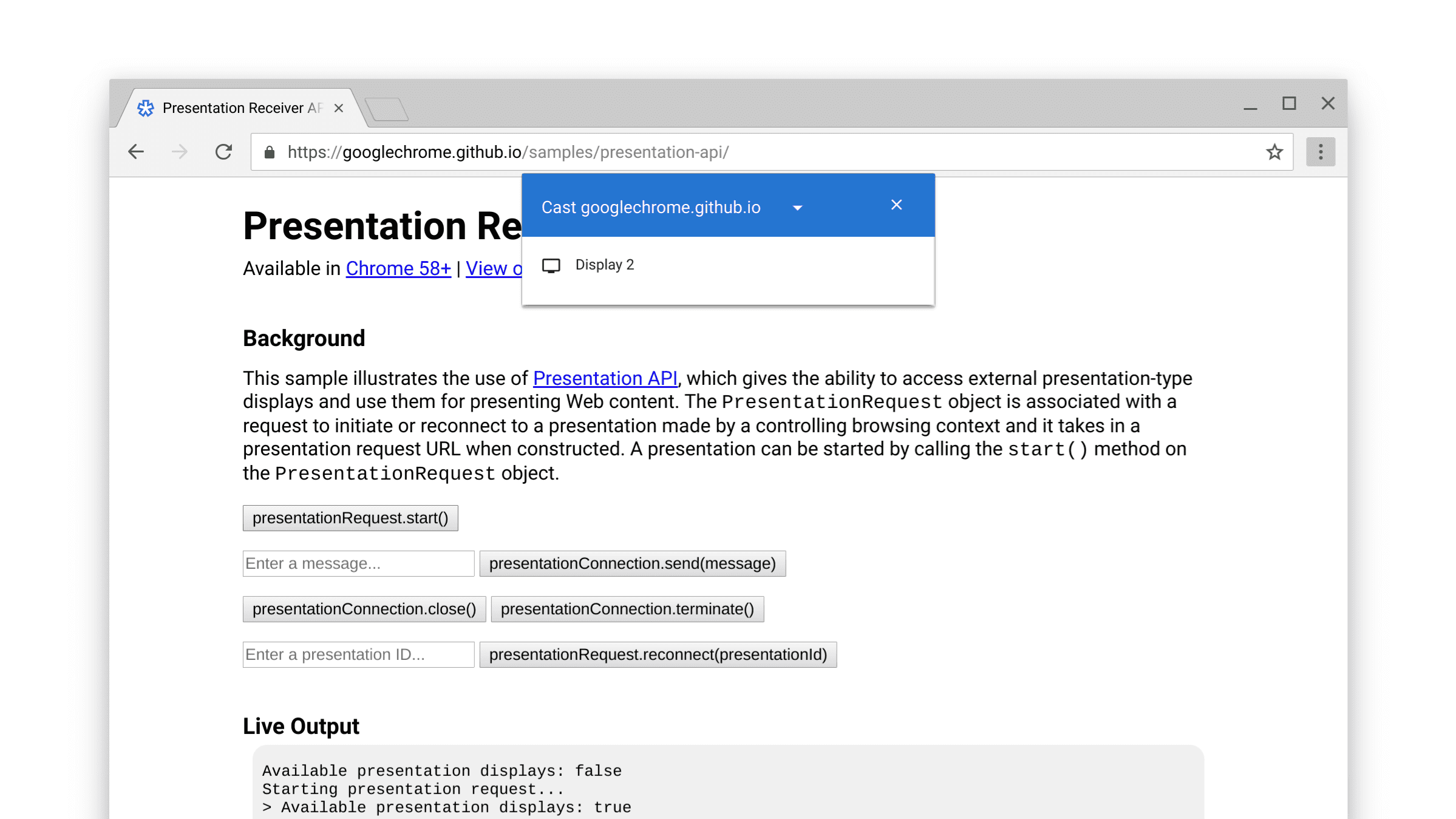
Promise が解決されると、PresentationRequest
オブジェクトの URL にあるウェブページが、選択したディスプレイに表示されます。これで完了です。
これで、さらに進んで、以下のように「close」イベントと「terminate」イベントをモニタリングできるようになりました。なお、presentationRequest.reconnect(presentationId)
を使用して「クローズされた」presentationConnection
に再接続できます。ここで、presentationId
は前の presentationRequest
オブジェクトの ID です。
function onCloseButtonClick() {
// Disconnect presentation connection but will allow reconnection.
presentationConnection.close();
}
presentationConnection.addEventListener('close', function() {
console.log('Connection closed.');
});
function onTerminateButtonClick() {
// Stop presentation connection for good.
presentationConnection.terminate();
}
presentationConnection.addEventListener('terminate', function() {
console.log('Connection terminated.');
});
ページとのやり取り
コントローラ ページ(先ほど作成したページ)とレシーバ ページ(PresentationRequest
オブジェクトに渡したページ)の間でメッセージを渡すにはどうすればよいでしょうか。
まず、以下に示すように、レシーバページで navigator.presentation.receiver.connectionList
を使用して既存の接続を取得し、受信接続をリッスンします。
// Receiver page
navigator.presentation.receiver.connectionList
.then(list => {
list.connections.map(connection => addConnection(connection));
list.addEventListener('connectionavailable', function(event) {
addConnection(event.connection);
});
});
function addConnection(connection) {
connection.addEventListener('message', function(event) {
console.log('Message: ' + event.data);
connection.send('Hey controller! I just received a message.');
});
connection.addEventListener('close', function(event) {
console.log('Connection closed!', event.reason);
});
}
接続でメッセージを受信すると、リッスン可能な「message」イベントが発生します。メッセージには、文字列、Blob、ArrayBuffer、ArrayBufferView を指定できます。送信は、コントローラ ページまたはレシーバページから connection.send(message)
を呼び出すだけで行えます。
// Controller page
function onSendMessageButtonClick() {
presentationConnection.send('Hello!');
}
presentationConnection.addEventListener('message', function(event) {
console.log('I just received ' + event.data + ' from the receiver.');
});
https://googlechrome.github.io/samples/presentation-api/ のサンプルで、動作を確認してみましょう。私と同じくらいお楽しみいただけると思います。
サンプルとデモ
この記事で使用した Chrome の公式サンプルをご覧ください。
Photowall のインタラクティブなデモもおすすめです。このウェブアプリを使用すると、複数のコントローラが連携して写真のスライドショーをプレゼンテーション ディスプレイに表示できます。コードは https://github.com/GoogleChromeLabs/presentation-api-samples から入手できます。
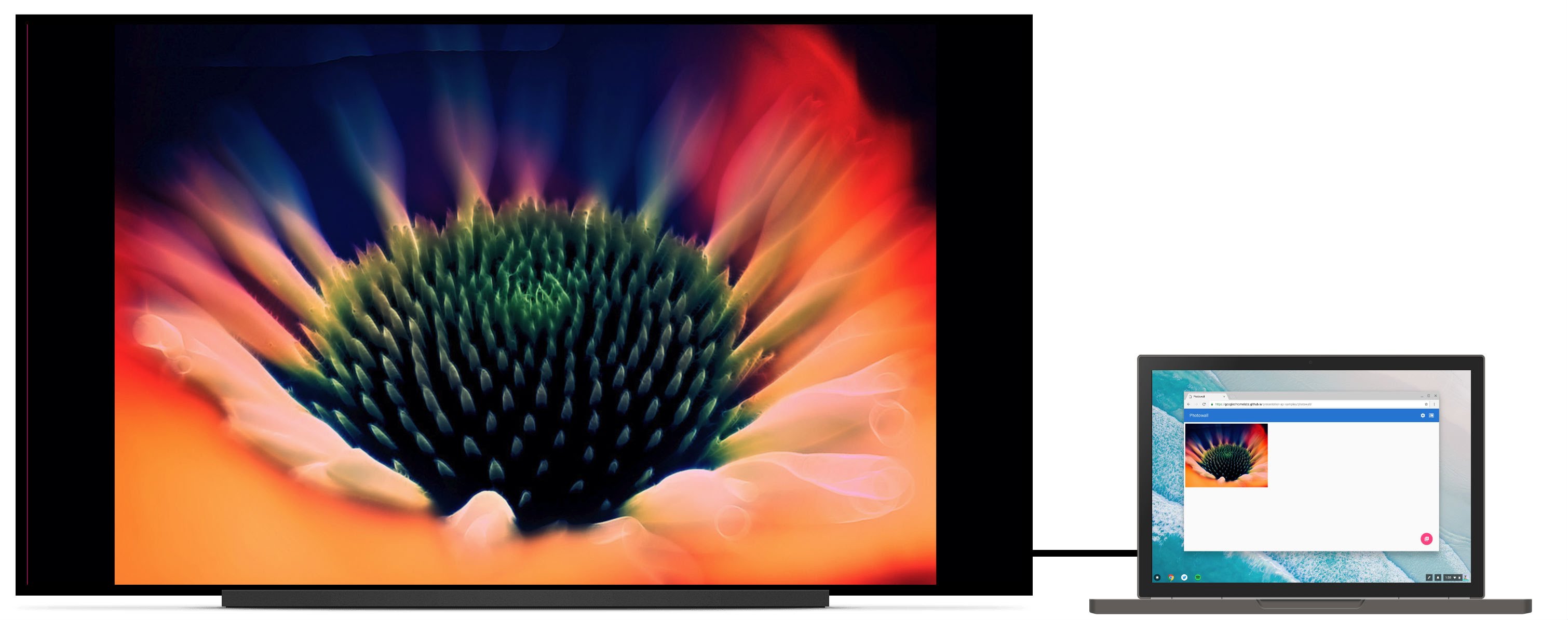
終わりに
Chrome には、ユーザーがウェブサイトにアクセスしたときにいつでも呼び出すことができる「キャスト」ブラウザ メニューがあります。このメニューのデフォルト表示を制御する場合は、前に作成したカスタム presentationRequest
オブジェクトに navigator.presentation.defaultRequest
を割り当てます。
// Make this presentation the default one when using the "Cast" browser menu.
navigator.presentation.defaultRequest = presentationRequest;
開発のヒント
レシーバーページを検査してデバッグするには、内部の chrome://inspect
ページに移動して [その他] を選択し、現在表示されている URL の横にある [検査] リンクをクリックします。
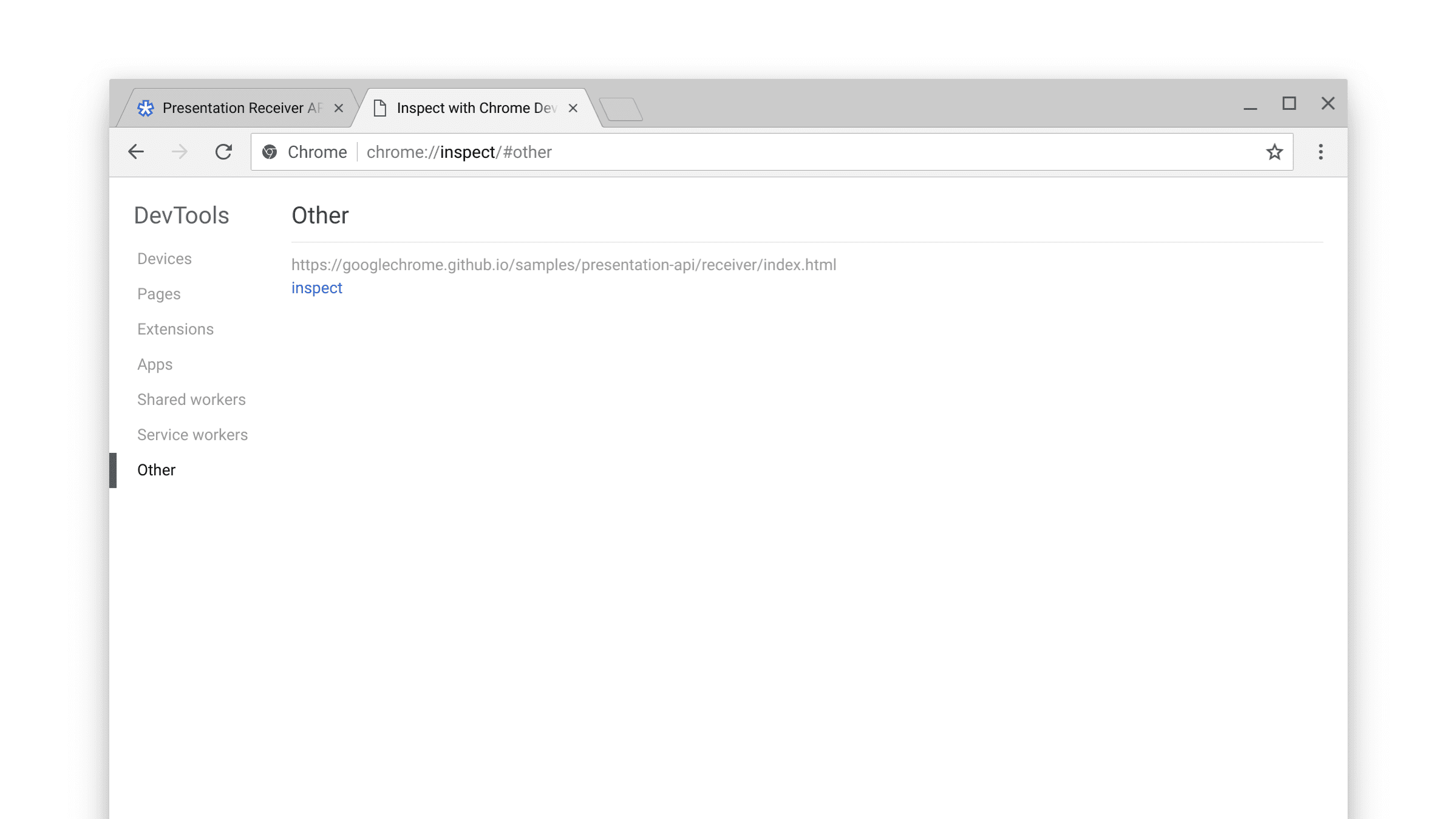
また、内部の検出/可用性プロセスの詳細については、内部の chrome://media-router-internals
ページもご確認ください。
次のステップ
Chrome 66 では、ChromeOS、Linux、Windows の各プラットフォームがサポートされています。Mac のサポートは今後提供される予定です。
リソース
- Chrome 機能のステータス: https://www.chromestatus.com/features#presentation%20api
- 実装のバグ: https://crbug.com/?q=component:Blink>PresentationAPI
- Presentation API の仕様: https://w3c.github.io/presentation-api/
- 仕様に関する問題: https://github.com/w3c/presentation-api/issues