Em 2021, a equipe do Chrome Aurora introduziu o componente de script para melhorar o carregamento de scripts de terceiros no Next.js. Desde o lançamento, ampliamos os recursos para facilitar e acelerar o carregamento de recursos de terceiros para os desenvolvedores.
Esta postagem do blog oferece uma visão geral dos recursos mais recentes que lançamos, principalmente a biblioteca @next/third-parties, bem como um resumo das iniciativas futuras no nosso cronograma.
Implicações de desempenho dos scripts de terceiros
41% de todas as solicitações de terceiros em sites do Next.js são scripts. Ao contrário de outros tipos de conteúdo, os scripts podem levar um tempo considerável para serem transferidos por download e executados, o que pode bloquear a renderização e atrasar a interatividade do usuário. Os dados do Chrome User Experience Report (CrUX) mostram que os sites Next.js que carregam mais scripts de terceiros têm taxas de aprovação mais baixas de Interaction to Next Paint (INP) e Largest Contentful Paint (LCP).
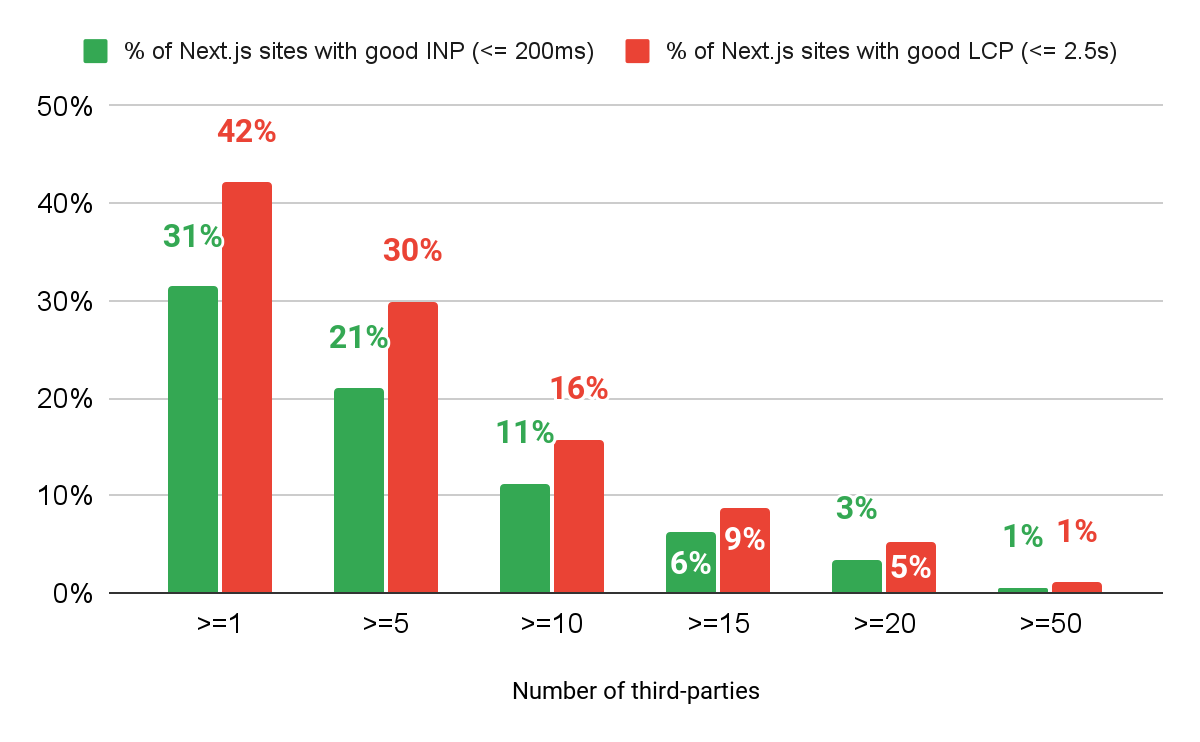
A correlação observada neste gráfico não implica causalidade. No entanto, os experimentos locais fornecem evidências adicionais de que os scripts de terceiros afetam significativamente a performance da página. Por exemplo, o gráfico abaixo compara várias métricas do Labs quando um contêiner do Gerenciador de tags do Google, que inclui 18 tags selecionadas aleatoriamente, é adicionado à Taxonomy, um exemplo de app Next.js conhecido.
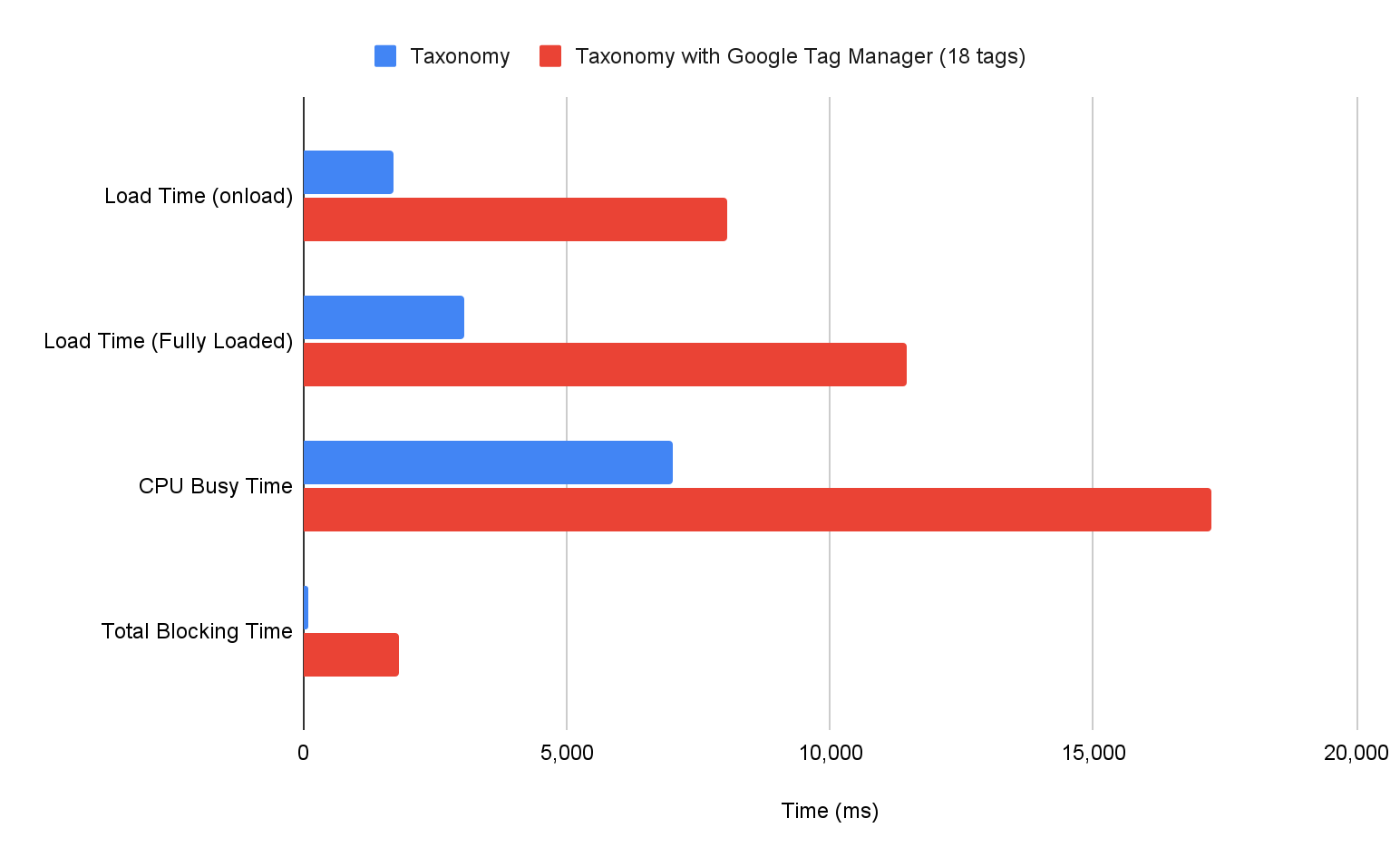
A documentação do WebPageTest oferece detalhes sobre como esses tempos são medidos. De relance, fica claro que todas essas métricas do laboratório são afetadas pelo contêiner do GTM. Por exemplo, o Tempo total de bloqueio (TBT, na sigla em inglês), um proxy de laboratório útil que se aproxima do INP, teve um aumento de quase 20 vezes.
Componente de script
Quando lançamos o componente <Script>
no Next.js, nos certificamos de apresentá-lo
por meio de uma API fácil de usar que se assemelha muito ao elemento <script>
tradicional. Com ele, os desenvolvedores podem colocar um script de terceiros em qualquer
componente do aplicativo, e o Next.js vai se encarregar de ordenar o
script depois que os recursos críticos forem carregados.
<!-- By default, script will load after page becomes interactive -->
<Script src="https://example.com/sample.js" />
<!-- Script is injected server-side and fetched before any page hydration occurs -->
<Script strategy=”beforeInteractive” src="https://example.com/sample.js" />
<!-- Script is fetched later during browser idle time -->
<Script strategy=”lazyOnload” src="https://example.com/sample.js" />
Dezenas de milhares de aplicativos Next.js, incluindo sites conhecidos como
Patreon, Target e
Notion, usam o componente <Script>
. Apesar da
eficácia, alguns desenvolvedores expressaram preocupação com os seguintes
assuntos:
- Onde colocar o componente
<Script>
em um app do Next.js, seguindo as diferentes instruções de instalação de diferentes provedores de terceiros (experiência do desenvolvedor). - Qual estratégia de carregamento é mais adequada para diferentes scripts de terceiros (experiência do usuário).
Para resolver essas duas questões, lançamos a @next/third-parties
, uma
biblioteca especializada que oferece um conjunto de componentes e utilitários otimizados
personalizados para terceiros conhecidos.
Experiência do desenvolvedor: como facilitar o gerenciamento de bibliotecas de terceiros
Muitos scripts de terceiros são usados em uma porcentagem significativa de sites Next.js, sendo o Gerenciador de tags do Google o mais popular, usado por 66% dos sites, respectivamente.
O @next/third-parties
é construído sobre o componente <Script>
com a introdução de wrappers de nível mais alto projetados para simplificar o uso desses
casos de uso comuns.
import { GoogleAnalytics } from "@next/third-parties/google";
export default function RootLayout({ children }) {
return (
<html lang="en">
<body>{children}</body>
<GoogleTagManager gtmId="GTM-XYZ" />
</html>
);
}
O Google Analytics, outro script de terceiros amplamente usado (52% dos sites do Next.js), também tem um componente dedicado.
import { GoogleAnalytics } from "@next/third-parties/google";
export default function RootLayout({ children }) {
return (
<html lang="en">
<body>{children}</body>
<GoogleAnalytics gaId="G-XYZ" />
</html>
);
}
@next/third-parties
simplifica o processo de carregamento de scripts usados com frequência,
mas também amplia nossa capacidade de desenvolver utilitários para outras categorias
de terceiros, como incorporações. Por exemplo, as incorporações do Google Maps e do YouTube são
usadas em
8%
e
4%
dos sites do Next.js, respectivamente. Também lançamos componentes para facilitar o
carregamento.
import { GoogleMapsEmbed } from "@next/third-parties/google";
import { YouTubeEmbed } from "@next/third-parties/google";
export default function Page() {
return (
<>
<GoogleMapsEmbed
apiKey="XYZ"
height={200}
width="100%"
mode="place"
q="Brooklyn+Bridge,New+York,NY"
/>
<YouTubeEmbed videoid="ogfYd705cRs" height={400} params="controls=0" />
</>
);
}
Experiência do usuário: como fazer com que as bibliotecas de terceiros carreguem mais rápido
Em um mundo perfeito, todas as bibliotecas de terceiros amplamente adotadas seriam totalmente otimizadas, tornando desnecessárias as abstrações que melhoram o desempenho delas. No entanto, até que isso se torne realidade, podemos tentar melhorar a experiência do usuário quando integrada a frameworks conhecidos, como o Next.js. Podemos testar diferentes técnicas de carregamento, garantir que os scripts sejam sequenciados da maneira certa e, por fim, compartilhar nosso feedback com provedores de terceiros para incentivar mudanças upstream.
Por exemplo, as incorporações do YouTube. Em que algumas implementações alternativas têm
um desempenho muito melhor do que a incorporação nativa. Atualmente, o componente <YouTubeEmbed>
exportado por @next/third-parties
usa
lite-youtube-embed, que,
quando demonstrado em uma comparação "Hello, World" Next.js, carrega de forma
consideravelmente mais rápida.
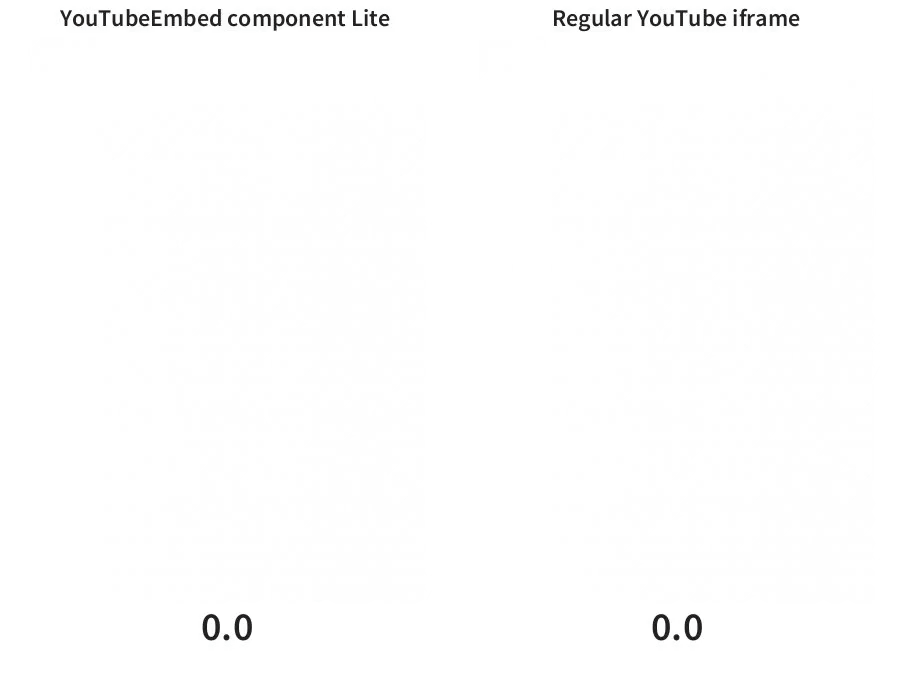
Da mesma forma, para o Google Maps, incluímos loading="lazy"
como um atributo padrão para
a incorporação, para garantir que o mapa seja carregado apenas quando estiver a uma certa distância da
janela de visualização. Isso pode parecer um atributo óbvio para incluir, especialmente
porque a documentação
do Google Maps o inclui no exemplo de snippet de código, mas apenas
45% dos sites do Next.js que incorporam o Google Maps estão usando loading="lazy"
.
Como executar scripts de terceiros em um worker da Web
Uma técnica avançada que estamos explorando no @next/third-parties
é facilitar
a transferência dos scripts de terceiros para um worker da Web. Popularizado por
bibliotecas como Partytown, isso pode reduzir
significativamente o impacto de scripts de terceiros na performance da página,
relocando-os totalmente fora da linha de execução principal.
O GIF animado a seguir mostra as variações nas tarefas longas e no tempo de bloqueio da linha de execução
principal ao aplicar diferentes estratégias <Script>
a um contêiner do GTM
em um site do Next.js. Embora a troca entre as opções de estratégia apenas
atrase o momento em que esses scripts são executados, a mudança para um worker da Web
elimina completamente o tempo deles na linha de execução principal.

Neste exemplo específico, a mudança da execução do contêiner do GTM e dos scripts de tag associados para um worker da Web reduziu o TBT em 92%.
Vale ressaltar que, se não for gerenciada com cuidado, essa técnica pode interromper
vários scripts de terceiros, dificultando a depuração. Nos próximos
meses, vamos validar se os componentes de terceiros oferecidos pelo
@next/third-parties
funcionam corretamente quando executados em um worker da Web. Se for o caso, vamos
trabalhar para oferecer uma maneira fácil e opcional para que os desenvolvedores usem essa
técnica.
Próximas etapas
No processo de desenvolvimento desse pacote, ficou evidente que era necessário
centralizar as recomendações de carregamento de terceiros para que outros frameworks
também pudessem se beneficiar das mesmas técnicas usadas. Isso nos levou a
criar o Third Party
Capital, uma biblioteca
que usa JSON para descrever técnicas de carregamento de terceiros, que atualmente
servem como base para @next/third-parties
.
Nas próximas etapas, vamos continuar nos concentrando em melhorar os componentes fornecidos para o Next.js e ampliar nossos esforços para incluir utilitários semelhantes em outros frameworks e plataformas de CMS. No momento, estamos em colaboração com os mantenedores do Nuxt e planejamos lançar utilitários de terceiros semelhantes adaptados ao ecossistema deles em breve.
Se uma das partes de terceiros que você usa no seu app Next.js tiver suporte para
@next/third-parties
,
instale o pacote
e teste. Queremos saber sua opinião no
GitHub.