One advantage of Custom Tabs is that they can be seamlessly integrated into your app. In this part of the Custom Tabs guide, you will learn how to change the appearance and behavior of a Custom Tab to match your app.
Set the color of the toolbar
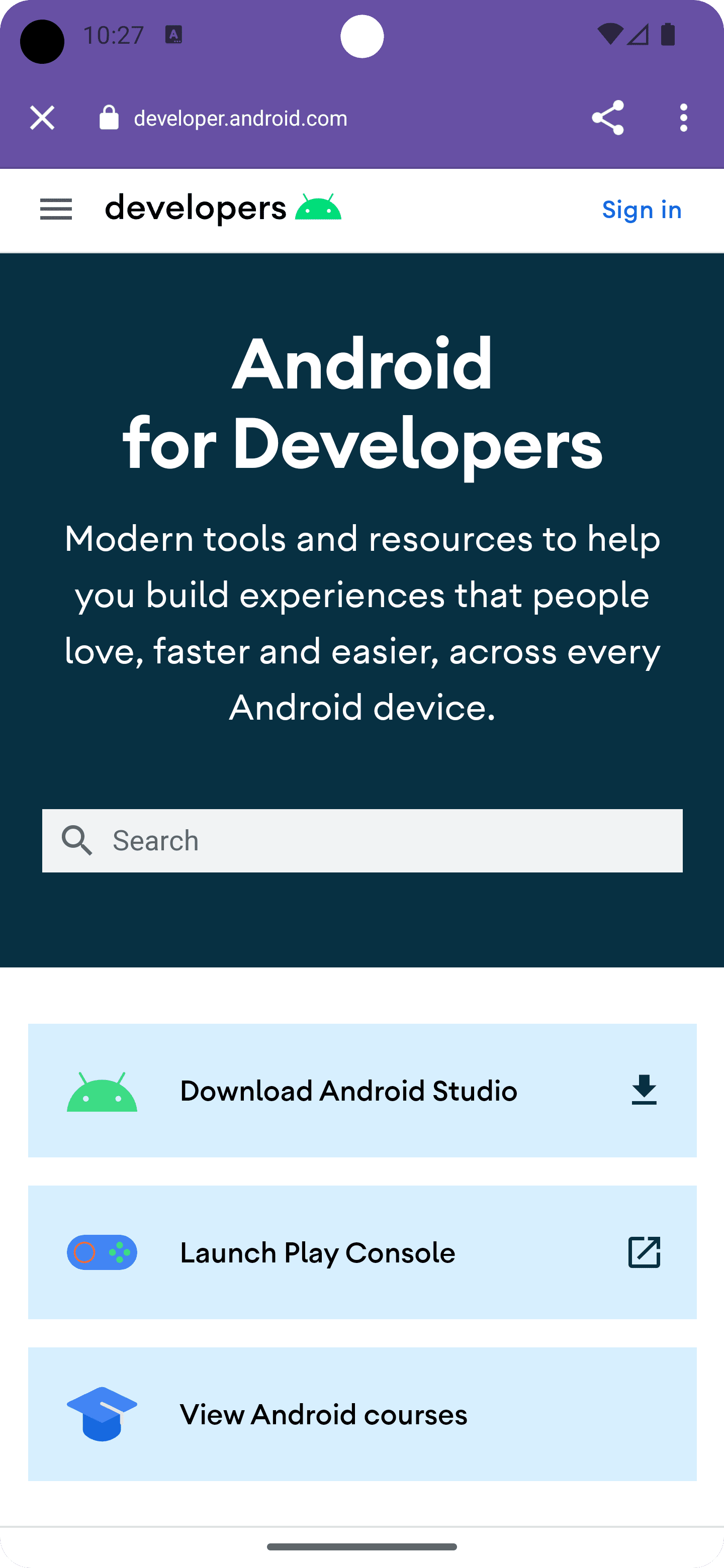
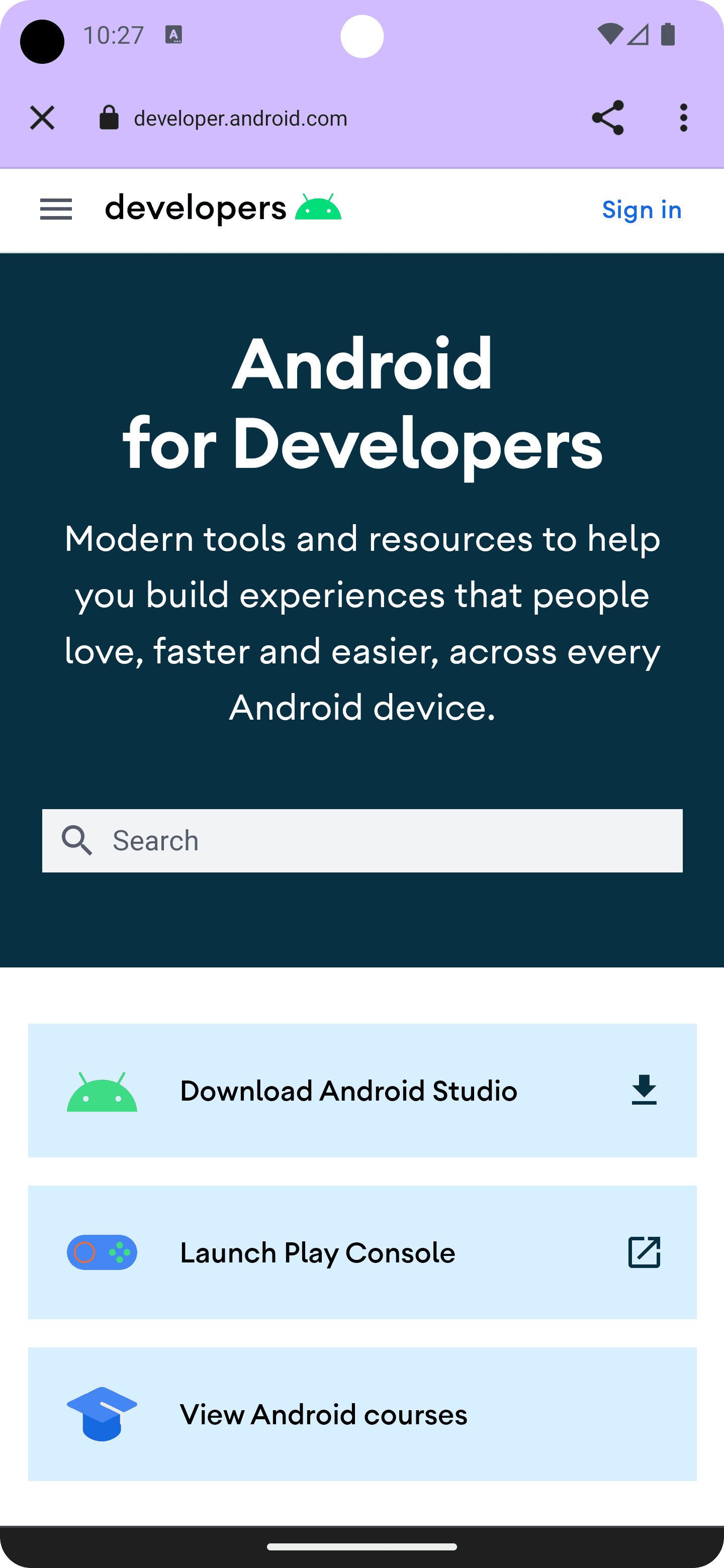
First, customizing the Custom Tab’s address bar to be consistent with your app's theme. The snippet below changes the default toolbar color by calling setDefaultColorSchemeParams()
. If your app also supports a dark color scheme, set it via .setColorSchemeParams(CustomTabsIntent.COLOR_SCHEME_DARK, …)
.
// get the current toolbar background color (this might work differently in your app)
@ColorInt int colorPrimaryLight = ContextCompat.getColor(MainActivity.this, R.color.md_theme_light_primary);
@ColorInt int colorPrimaryDark = ContextCompat.getColor(MainActivity.this, R.color.md_theme_dark_primary);
CustomTabsIntent intent = new CustomTabsIntent.Builder()
// set the default color scheme
.setDefaultColorSchemeParams(new CustomTabColorSchemeParams.Builder()
.setToolbarColor(colorPrimaryLight)
.build())
// set the alternative dark color scheme
.setColorSchemeParams(CustomTabsIntent.COLOR_SCHEME_DARK, new CustomTabColorSchemeParams.Builder()
.setToolbarColor(colorPrimaryDark)
.build())
.build();
The toolbar now has custom background and foreground colors.
Configure custom enter and exit animation
Next, you can make launching (and leaving) a Custom Tab experience in your app more seamless, by defining custom start and exit animations using setStartAnimation
and setExitAnimation
:
CustomTabsIntent intent = new CustomTabsIntent.Builder()
…
.setStartAnimations(MainActivity.this, R.anim.slide_in_right, R.anim.slide_out_left)
.setExitAnimations(MainActivity.this, android.R.anim.slide_in_left, android.R.anim.slide_out_right)
.build();
Further customizations: title, autohide AppBar, and custom close icon
There are a few more things you can do to adjust the UI of a Custom Tab to your needs.
- Hide the URL bar on scroll to give the user more space to explore web content using
setUrlBarHidingEnabled()(true)
. - Show the document title instead of the URL via
setShowTitle()(true)
. - Customize the close button to match the user flow in your app, for example, by showing a back arrow instead of the default
X
icon):setCloseButtonIcon()(myCustomCloseIcon)
.
These are all optional, but they can improve the Custom Tab experience in your app.
Bitmap myCustomCloseIcon = getDrawable(R.drawable.ic_baseline_arrow_back_24));
CustomTabsIntent intent = new CustomTabsIntent.Builder()
…
.setUrlBarHidingEnabled(true)
.setShowTitle(true)
.setCloseButtonIcon(toBitmap(myCustomCloseIcon))
.build();
Setting a custom referrer
You can set your app as the referrer when launching your Custom Tab. This way you can let websites know where their traffic is coming from.
CustomTabsIntent customTabsIntent = new CustomTabsIntent.Builder().build()
customTabsIntent.intent.putExtra(Intent.EXTRA_REFERRER,
Uri.parse("android-app://" + context.getPackageName()));