對網頁開發人員來說,WebGPU 是一種網路圖形 API,可以提供統一而快速的使用體驗 提供 GPU 存取權WebGPU 提供現代硬體功能,並允許轉譯 和運算作業,類似於 Direct3D 12、 Metal 和 Vulkan。
事實是,這個故事不夠完整。WebGPU 是互助合作的成果 ,包括 Apple、Google、Intel、Mozilla 和 Microsoft。其中有些確實 它的特點是 JavaScript API 但跨平台的圖形 API,適用於整個生態系統 (而非網路) 的開發人員。
為了滿足主要用途 推出。不過,另一個重大 webgpu.h C 也能使用 Google Cloud CLI 或 Compute Engine API這個 C 標頭檔案列出所有可用程序和資料結構 WebGPU 的根源。做為各平台通用的硬體抽象層 提供一致的介面,協助您建構特定平台的應用程式 在不同平台上放送相關廣告
在這份文件中,您會瞭解如何使用 WebGPU 編寫在網路和特定平台上執行的小型 C++ 應用程式。劇透警示:你會在瀏覽器視窗與桌面視窗中看到相同的紅色三角形,只要對程式碼集進行微幅調整即可。
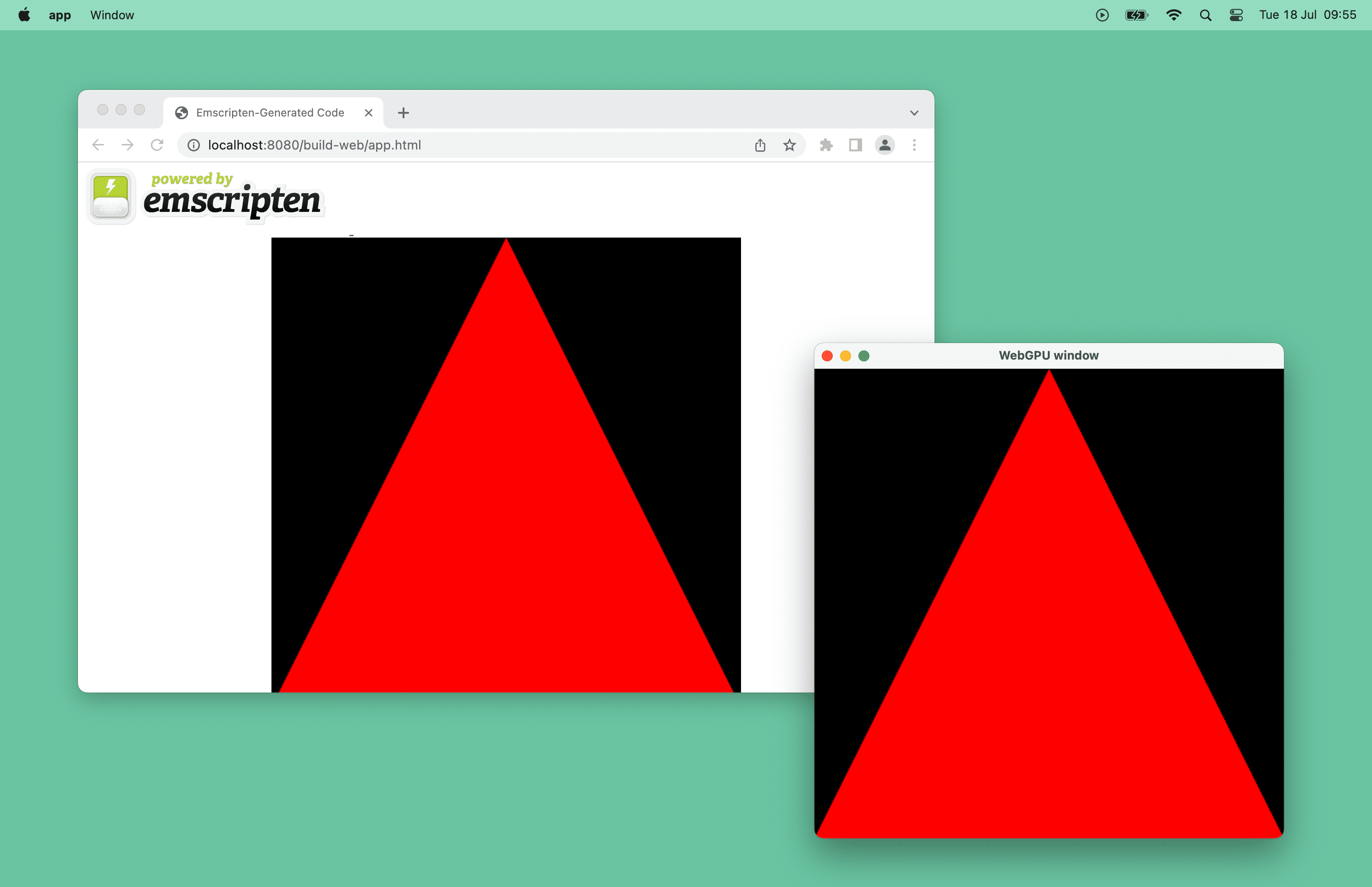
運作方式
如要查看完成的應用程式,請參閱 WebGPU 跨平台應用程式存放區。
這款應用程式是極簡風的 C++ 範例,說明如何使用 WebGPU 從單一程式碼集建構電腦版和網頁應用程式。基本上,它會透過名為 webgpu_cpp.h 的 C++ 包裝函式來使用 WebGPU 的 webgpu.h 做為各平台通用的硬體抽象層。
在網頁上,應用程式是以 Emscripten 為基礎建構而成,而該通訊協定在 JavaScript API 上方設有實作 webgpu.h 的繫結。在 macOS 或 Windows 等特定平台上,這個專案可根據 Chromium 的跨平台 WebGPU 實作 Dawn 來建構。值得一提的是 wgpu-native 這個 webgpu.h 的 Rust 實作項目,但這份文件並未使用。
開始使用
首先,您需要 C++ 編譯器和 CMake,以標準方式處理跨平台建構作業。在專用資料夾中建立
main.cpp
來源檔案和 CMakeLists.txt
建構檔案。
main.cpp
檔案目前應包含空白的 main()
函式。
int main() {}
CMakeLists.txt
檔案含有專案的基本資訊。最後一行的指定執行檔名稱為「app」,原始碼為 main.cpp
cmake_minimum_required(VERSION 3.13) # CMake version check
project(app) # Create project "app"
set(CMAKE_CXX_STANDARD 20) # Enable C++20 standard
add_executable(app "main.cpp")
執行 cmake -B build
以在「build/」中建立建構檔案子資料夾和 cmake --build build
,實際建構應用程式並產生可執行檔。
# Build the app with CMake.
$ cmake -B build && cmake --build build
# Run the app.
$ ./build/app
應用程式可以執行,但目前還沒有輸出內容,因為您需要設法在畫面上繪製內容。
開啟黎明
如要繪製三角形,您可以利用 Chromium 的跨平台 WebGPU 實作 Dawn。這包括用於在螢幕上繪圖的 GLFW C++ 程式庫。下載 Dawn 的方式之一是將其新增為存放區的 git 子模組。下列指令會在「dawn/」中擷取子資料夾
$ git init
$ git submodule add https://dawn.googlesource.com/dawn
接著,附加至 CMakeLists.txt
檔案,如下所示:
- CMake
DAWN_FETCH_DEPENDENCIES
選項會擷取所有 Dawn 依附元件。 - 目標會包含
dawn/
子資料夾。 - 應用程式將依附於
dawn::webgpu_dawn
、glfw
和webgpu_glfw
目標,方便您日後在main.cpp
檔案中使用。
…
set(DAWN_FETCH_DEPENDENCIES ON)
add_subdirectory("dawn" EXCLUDE_FROM_ALL)
target_link_libraries(app PRIVATE dawn::webgpu_dawn glfw webgpu_glfw)
開啟視窗
現在可以使用 Dawn 了,請使用 GLFW 在螢幕上繪圖。為方便起見,webgpu_glfw
包含這個程式庫,可讓您編寫適用於視窗管理不受平台影響的程式碼。
開啟名為「WebGPU 視窗」的視窗。如果解析度為 512x512,請按照下列方式更新 main.cpp
檔案。請注意,這裡 glfwWindowHint()
是用來要求沒有特定圖形 API 初始化。
#include <GLFW/glfw3.h>
const uint32_t kWidth = 512;
const uint32_t kHeight = 512;
void Start() {
if (!glfwInit()) {
return;
}
glfwWindowHint(GLFW_CLIENT_API, GLFW_NO_API);
GLFWwindow* window =
glfwCreateWindow(kWidth, kHeight, "WebGPU window", nullptr, nullptr);
while (!glfwWindowShouldClose(window)) {
glfwPollEvents();
// TODO: Render a triangle using WebGPU.
}
}
int main() {
Start();
}
重新建構應用程式並照常執行,會產生空白視窗。你正在進步中!
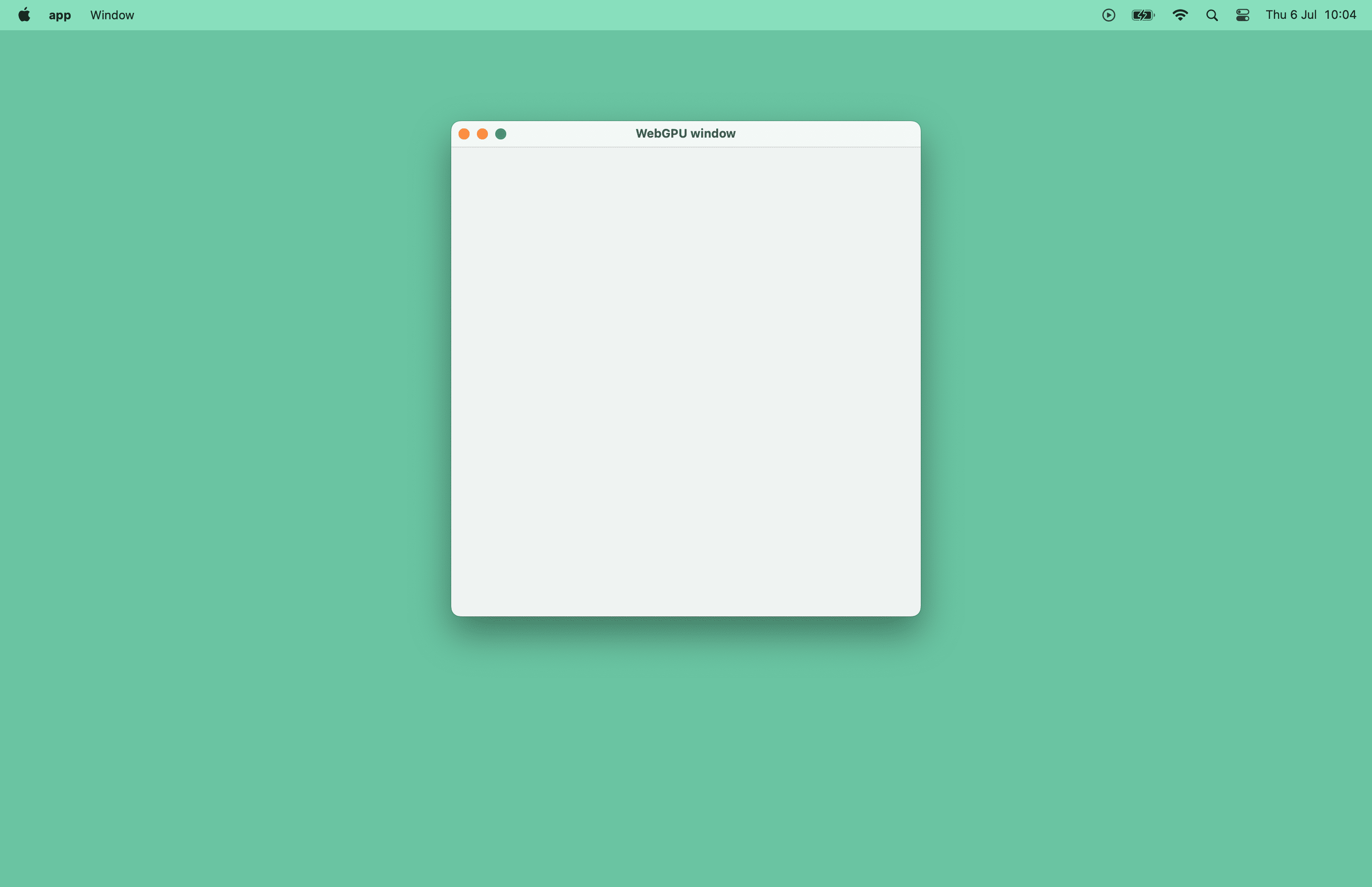
取得 GPU 裝置
在 JavaScript 中,navigator.gpu
是存取 GPU 的進入點。在 C++ 中,您必須手動建立用於相同用途的 wgpu::Instance
變數。為了方便起見,請在 main.cpp
檔案頂端宣告 instance
,然後在 main()
中呼叫 wgpu::CreateInstance()
。
…
#include <webgpu/webgpu_cpp.h>
wgpu::Instance instance;
…
int main() {
instance = wgpu::CreateInstance();
Start();
}
由於 JavaScript API 的形狀, GPU 是以非同步的方式存取 GPU。在 C++ 中,建立稱為 GetAdapter()
和 GetDevice()
的兩個輔助函式,分別傳回包含 wgpu::Adapter
和 wgpu::Device
的回呼函式。
#include <iostream>
…
void GetAdapter(void (*callback)(wgpu::Adapter)) {
instance.RequestAdapter(
nullptr,
[](WGPURequestAdapterStatus status, WGPUAdapter cAdapter,
const char* message, void* userdata) {
if (status != WGPURequestAdapterStatus_Success) {
exit(0);
}
wgpu::Adapter adapter = wgpu::Adapter::Acquire(cAdapter);
reinterpret_cast<void (*)(wgpu::Adapter)>(userdata)(adapter);
}, reinterpret_cast<void*>(callback));
}
void GetDevice(void (*callback)(wgpu::Device)) {
adapter.RequestDevice(
nullptr,
[](WGPURequestDeviceStatus status, WGPUDevice cDevice,
const char* message, void* userdata) {
wgpu::Device device = wgpu::Device::Acquire(cDevice);
device.SetUncapturedErrorCallback(
[](WGPUErrorType type, const char* message, void* userdata) {
std::cout << "Error: " << type << " - message: " << message;
},
nullptr);
reinterpret_cast<void (*)(wgpu::Device)>(userdata)(device);
}, reinterpret_cast<void*>(callback));
}
為方便存取,請在 main.cpp
檔案頂端宣告 wgpu::Adapter
和 wgpu::Device
兩個變數。更新 main()
函式以呼叫 GetAdapter()
,並將結果回呼指派給 adapter
,然後呼叫 GetDevice()
,並將結果回呼指派給 device
,再呼叫 Start()
。
wgpu::Adapter adapter;
wgpu::Device device;
…
int main() {
instance = wgpu::CreateInstance();
GetAdapter([](wgpu::Adapter a) {
adapter = a;
GetDevice([](wgpu::Device d) {
device = d;
Start();
});
});
}
繪製三角形
瀏覽器會負責處理,因此不會在 JavaScript API 中公開交換鏈。在 C++ 中,您必須手動建立。為方便起見,再次在 main.cpp
檔案的頂端宣告 wgpu::Surface
變數。在 Start()
中建立 GLFW 視窗後,呼叫實用的 wgpu::glfw::CreateSurfaceForWindow()
函式來建立 wgpu::Surface
(類似 HTML 畫布),然後在 InitGraphics()
中呼叫新的輔助 ConfigureSurface()
函式,以進行設定。您還需要呼叫 surface.Present()
,才能在 when 迴圈中顯示下一個紋理。由於尚未發生任何轉譯作業,這項操作不會造成明顯影響。
#include <webgpu/webgpu_glfw.h>
…
wgpu::Surface surface;
wgpu::TextureFormat format;
void ConfigureSurface() {
wgpu::SurfaceCapabilities capabilities;
surface.GetCapabilities(adapter, &capabilities);
format = capabilities.formats[0];
wgpu::SurfaceConfiguration config{
.device = device,
.format = format,
.width = kWidth,
.height = kHeight};
surface.Configure(&config);
}
void InitGraphics() {
ConfigureSurface();
}
void Render() {
// TODO: Render a triangle using WebGPU.
}
void Start() {
…
surface = wgpu::glfw::CreateSurfaceForWindow(instance, window);
InitGraphics();
while (!glfwWindowShouldClose(window)) {
glfwPollEvents();
Render();
surface.Present();
instance.ProcessEvents();
}
}
現在,建議使用下列程式碼建立轉譯管道。為方便存取,請在 main.cpp
檔案頂端宣告 wgpu::RenderPipeline
變數,並呼叫 InitGraphics()
中的輔助函式 CreateRenderPipeline()
。
wgpu::RenderPipeline pipeline;
…
const char shaderCode[] = R"(
@vertex fn vertexMain(@builtin(vertex_index) i : u32) ->
@builtin(position) vec4f {
const pos = array(vec2f(0, 1), vec2f(-1, -1), vec2f(1, -1));
return vec4f(pos[i], 0, 1);
}
@fragment fn fragmentMain() -> @location(0) vec4f {
return vec4f(1, 0, 0, 1);
}
)";
void CreateRenderPipeline() {
wgpu::ShaderModuleWGSLDescriptor wgslDesc{};
wgslDesc.code = shaderCode;
wgpu::ShaderModuleDescriptor shaderModuleDescriptor{
.nextInChain = &wgslDesc};
wgpu::ShaderModule shaderModule =
device.CreateShaderModule(&shaderModuleDescriptor);
wgpu::ColorTargetState colorTargetState{.format = format};
wgpu::FragmentState fragmentState{.module = shaderModule,
.targetCount = 1,
.targets = &colorTargetState};
wgpu::RenderPipelineDescriptor descriptor{
.vertex = {.module = shaderModule},
.fragment = &fragmentState};
pipeline = device.CreateRenderPipeline(&descriptor);
}
void InitGraphics() {
…
CreateRenderPipeline();
}
最後,透過呼叫每個影格的 Render()
函式,將轉譯指令傳送至 GPU。
void Render() {
wgpu::SurfaceTexture surfaceTexture;
surface.GetCurrentTexture(&surfaceTexture);
wgpu::RenderPassColorAttachment attachment{
.view = surfaceTexture.texture.CreateView(),
.loadOp = wgpu::LoadOp::Clear,
.storeOp = wgpu::StoreOp::Store};
wgpu::RenderPassDescriptor renderpass{.colorAttachmentCount = 1,
.colorAttachments = &attachment};
wgpu::CommandEncoder encoder = device.CreateCommandEncoder();
wgpu::RenderPassEncoder pass = encoder.BeginRenderPass(&renderpass);
pass.SetPipeline(pipeline);
pass.Draw(3);
pass.End();
wgpu::CommandBuffer commands = encoder.Finish();
device.GetQueue().Submit(1, &commands);
}
現在使用 CMake 重新建構應用程式並加以執行,會導致視窗中長久的紅色三角形!休息一下,你值得好好休息。
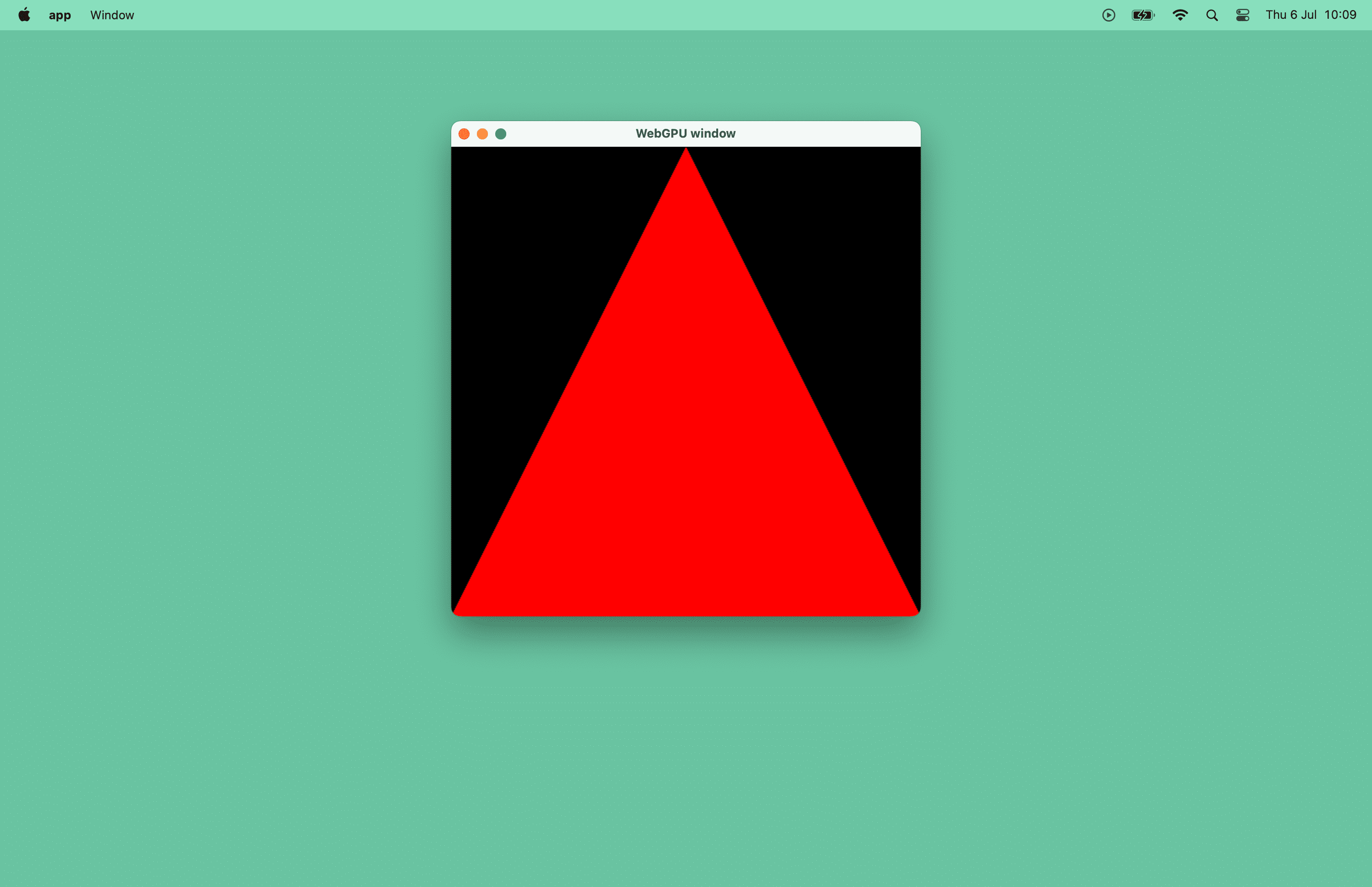
編譯至 WebAssembly
現在,讓我們來看看您最少必須進行哪些變更,才能調整現有的程式碼集,以便在瀏覽器視窗中繪製這個紅色三角形。同樣的,應用程式是以 Emscripten 為基礎建構而成;Emscripten 這項工具可用於將 C/C++ 程式編譯至 WebAssembly,後者俱備在 JavaScript API 上實作 webgpu.h 的繫結。
更新 CMake 設定
安裝 Emscripten 後,請按照下列步驟更新 CMakeLists.txt
建構檔案。
醒目顯示的程式碼是唯一需要變更的項目。
set_target_properties
可用來自動新增「html」副檔名為目標檔案。也就是說,您必須產生「app.html」檔案。- 如要在 Emscripten 中啟用 WebGPU 支援,必須要有「
USE_WEBGPU
」應用程式連結選項。如果沒有這個設定檔,main.cpp
檔案將無法存取webgpu/webgpu_cpp.h
檔案。 - 這裡也需要
USE_GLFW
應用程式連結選項,以便重複使用 GLFW 程式碼。
cmake_minimum_required(VERSION 3.13) # CMake version check
project(app) # Create project "app"
set(CMAKE_CXX_STANDARD 20) # Enable C++20 standard
add_executable(app "main.cpp")
if(EMSCRIPTEN)
set_target_properties(app PROPERTIES SUFFIX ".html")
target_link_options(app PRIVATE "-sUSE_WEBGPU=1" "-sUSE_GLFW=3")
else()
set(DAWN_FETCH_DEPENDENCIES ON)
add_subdirectory("dawn" EXCLUDE_FROM_ALL)
target_link_libraries(app PRIVATE dawn::webgpu_dawn glfw webgpu_glfw)
endif()
更新程式碼
在 Emscripten 中,建立 wgpu::surface
需要 HTML 畫布元素。在此情況下,請呼叫 instance.CreateSurface()
並指定 #canvas
選取器,使其符合 Emscripten 產生的 HTML 畫布元素。
請呼叫 emscripten_set_main_loop(Render)
,確保呼叫 Render()
函式時使用的是適合瀏覽器和監控器的正確流暢速率,而非使用迴圈迴圈。
#include <GLFW/glfw3.h>
#include <webgpu/webgpu_cpp.h>
#include <iostream>
#if defined(__EMSCRIPTEN__)
#include <emscripten/emscripten.h>
#else
#include <webgpu/webgpu_glfw.h>
#endif
void Start() {
if (!glfwInit()) {
return;
}
glfwWindowHint(GLFW_CLIENT_API, GLFW_NO_API);
GLFWwindow* window =
glfwCreateWindow(kWidth, kHeight, "WebGPU window", nullptr, nullptr);
#if defined(__EMSCRIPTEN__)
wgpu::SurfaceDescriptorFromCanvasHTMLSelector canvasDesc{};
canvasDesc.selector = "#canvas";
wgpu::SurfaceDescriptor surfaceDesc{.nextInChain = &canvasDesc};
surface = instance.CreateSurface(&surfaceDesc);
#else
surface = wgpu::glfw::CreateSurfaceForWindow(instance, window);
#endif
InitGraphics();
#if defined(__EMSCRIPTEN__)
emscripten_set_main_loop(Render, 0, false);
#else
while (!glfwWindowShouldClose(window)) {
glfwPollEvents();
Render();
surface.Present();
instance.ProcessEvents();
}
#endif
}
使用 Emscripten 建構應用程式
如要使用 Emscripten 建構應用程式,只需在前面加上 magical emcmake
殼層指令碼 cmake
指令即可。這次,請在 build-web
子資料夾中產生應用程式,並啟動 HTTP 伺服器。最後,開啟瀏覽器並前往 build-web/app.html
。
# Build the app with Emscripten.
$ emcmake cmake -B build-web && cmake --build build-web
# Start a HTTP server.
$ npx http-server
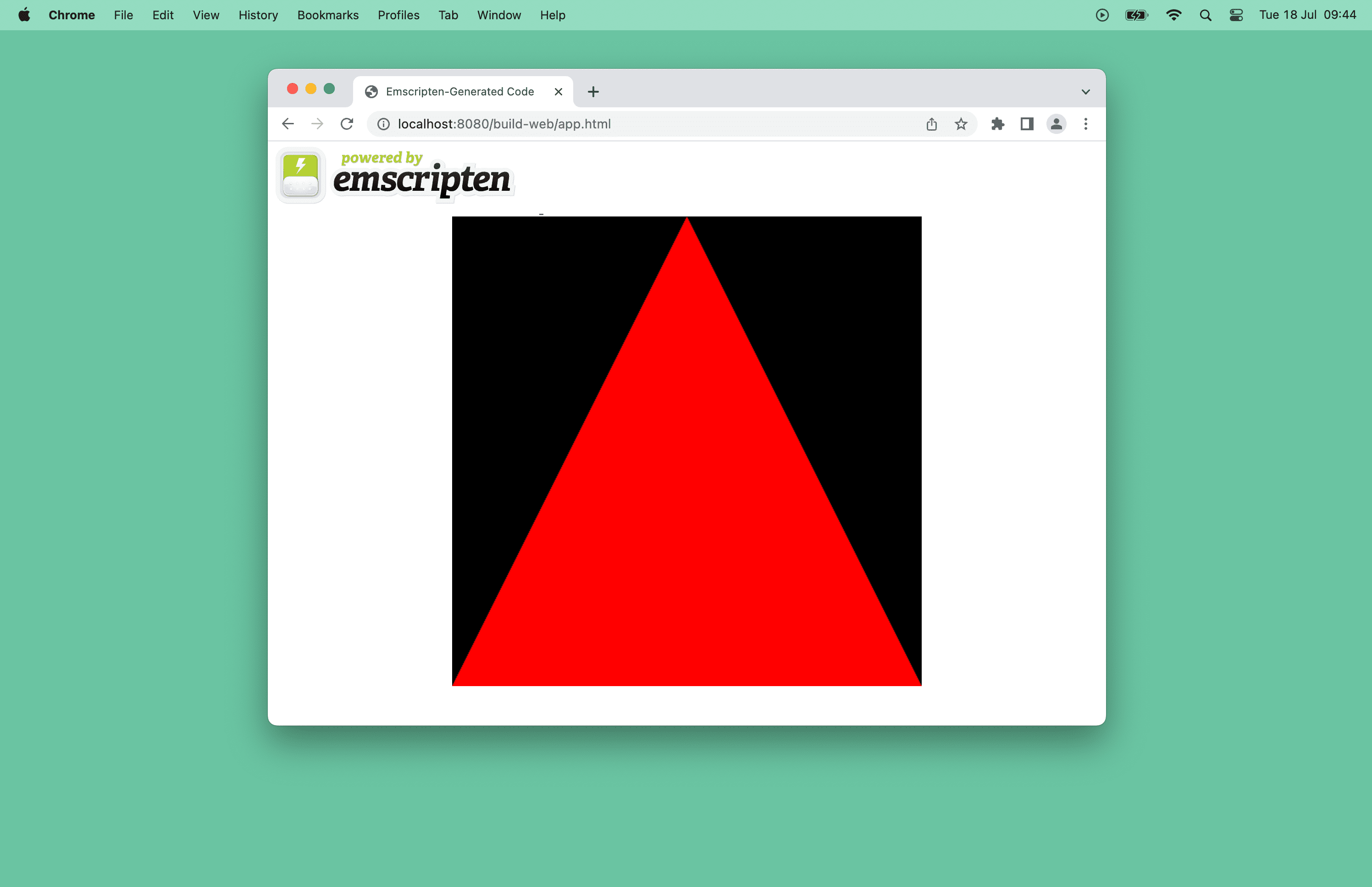
後續步驟
以下是日後推出的版本:
- 改善 webgpu.h 和 webgpu_cpp.h API 的穩定性。
- Dawn 初步支援 Android 和 iOS。
在此期間,你也可以提出 Emscripten 的 WebGPU 問題和 Dawn 問題,包括提出建議和問題。
資源
歡迎探索這個應用程式的原始碼。
如要進一步瞭解如何使用 WebGPU 在 C++ 中從頭開始建立原生 3D 應用程式,請參閱 瞭解 C++ 適用的 WebGPU 說明文件和 Dawn 原生 WebGPU 範例。
如果您對 Rust 感興趣,也可以探索以 WebGPU 為基礎的 wgpu 圖形程式庫。觀看 hello-triangle 示範
特別銘謝
本文評論者為 Corentin Wallez、Kai Ninomiya 和 Rachel Andrew。
相片來源:Marc-Olivier Jodoin,來源為 Unsplash。