Chrome 128 和 129 推出了 WebAuthn 的全新功能, 建構密碼金鑰式驗證系統。
- 提示:提示可讓信賴方 (RP) 進一步控管 WebAuthn 使用者介面。這對於企業使用者來說特別實用 ,才能使用安全金鑰
- 相關來源要求:有相關來源 要求,RP 可以讓密碼金鑰在多個網域上有效。如果您擁有多個 現在,您可以讓使用者在您的不同網站上重複使用自己的密碼金鑰, 減少登入過程中的困擾
- JSON 序列化:JSON 序列化 API 可讓您透過編碼和解碼選項來簡化 RP 的前端程式碼,以及 將憑證傳遞給 WebAuthn API,以及從 WebAuthn API 傳遞的憑證。
提示
使用 hints
時,依賴方 (RP) 現在可以指定使用者介面偏好設定來建立
使用密碼金鑰或密碼金鑰驗證。
過去,RP 要限制使用者
建立密碼金鑰或進行驗證
authenticatorSelection.authenticatorAttachment
:用於指定 "platform"
或
"cross-platform"
。他們分別將驗證器限制在一個平台
驗證器
或漫遊
驗證器。
使用 hints
時,這個規格可以更有彈性。
受限方可以在 PublicKeyCredentialCreationOptions
中使用選用的 hints
,或
PublicKeyCredentialRequestOptions
可指定 "security-key"
"client-device"
和 "hybrid"
,以陣列中的偏好設定順序排列。
以下範例是偏好憑證建立要求
以 "security-key"
做為提示的 "cross-platform"
驗證器。這告訴
Chrome 會為企業使用者顯示以安全金鑰為主的 UI。
const credential = await navigator.credentials.create({
publicKey: {
challenge: *****,
hints: ['security-key'],
authenticatorSelection: {
authenticatorAttachment: 'cross-platform'
}
}
});
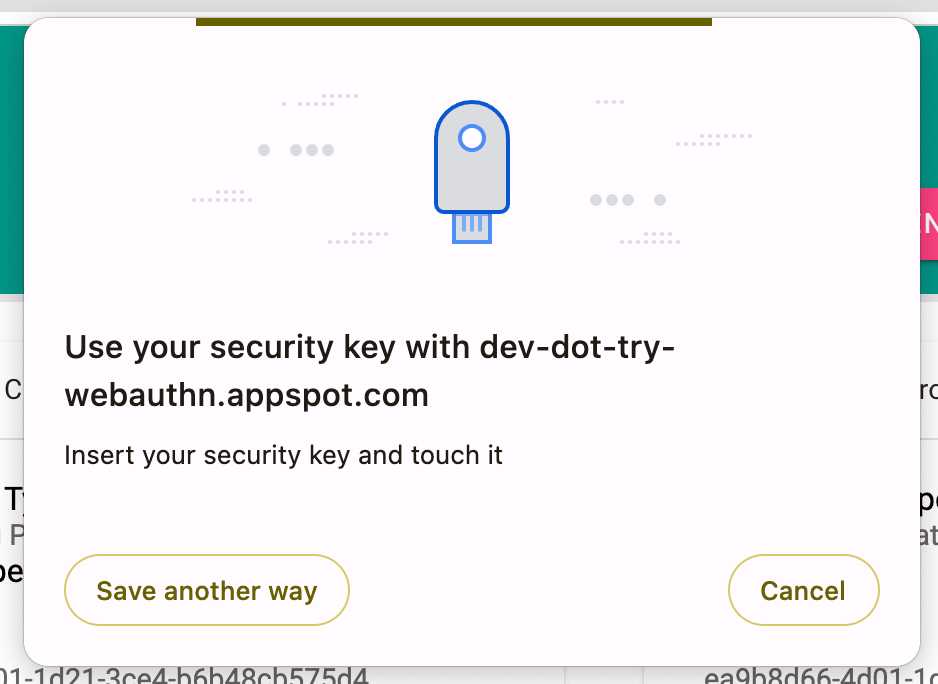
當 RP 想優先執行跨裝置驗證時,可以
傳送偏好 "cross-platform"
驗證器的驗證要求
以 "hybrid"
作為提示
const credential = await navigator.credentials.create({
publicKey: {
challenge: *****,
residentKey: true,
hints: ['hybrid']
authenticatorSelection: {
authenticatorAttachment: 'cross-platform'
}
}
});
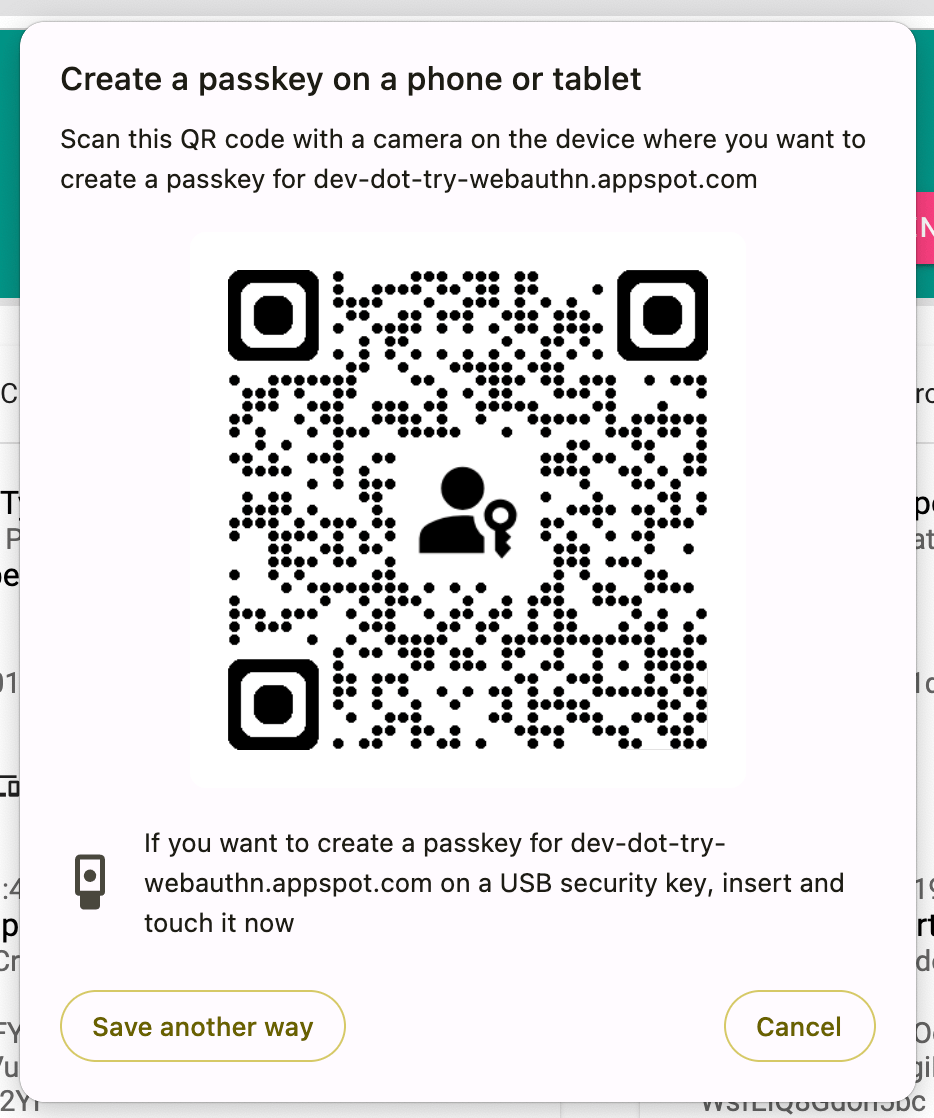
相關來源要求
有相關來源 要求,受限方可以 方便您在多個網域中使用密碼金鑰建立集中式登入 優質服務,以及使用聯合通訊協定 大部分的網站不過,如果您擁有多個網域且無法建立聯盟 相關的來源或許是解決問題的方法
所有 WebAuthn 要求都必須指定依賴方 ID (RP ID) 和所有密碼金鑰
都與單一受限方 ID 相關聯一般來說,來源只能指定
依據網域和 RP ID,因此在此例中,www.example.co.uk
可以指定
受限方 ID 為 example.co.uk
,但不是 example.com
。具有相關來源
要求,可透過擷取已知的 JSON 檔案來驗證已聲明的 RP ID
位於目標網域的 /.well-known/webauthn
。所以 example.co.uk
(和 example.in
、example.de
等) 都可以使用
如果 example.com
以下列格式指定位置,則為 example.com
:
網址:https://example.com/.well-known/webauthn
{
"origins": [
"https://example.co.uk",
"https://example.de",
"https://example.sg",
"https://example.net",
"https://exampledelivery.com",
"https://exampledelivery.co.uk",
"https://exampledelivery.de",
"https://exampledelivery.sg",
"https://myexamplerewards.com",
"https://examplecars.com"
]
}
如要瞭解如何設定相關來源要求,請參閱「允許在 具有相關來源的網站 要求。
JSON 序列化
WebAuthn 要求和回應物件有多個欄位,且包含原始 ArrayBuffer 中的二進位資料,例如憑證 ID、使用者 ID 或驗證方式。 如果網站要使用 JSON 來將這項資料與伺服器交換,二進位檔是 資料必須先編碼,例如使用 Base64URL 編碼這為無謂 如果開發人員想開始在網站上使用密碼金鑰,則變得相當複雜。
WebAuthn 現在提供可剖析的 API
PublicKeyCredentialCreationOptions
敬上
和
PublicKeyCredentialRequestOptions
WebAuthn 要求物件直接從 JSON 取得,還能將
PublicKeyCredential
直接寫入 JSON 中所有包含原始二進位的 ArrayBuffer 值欄位
資料會自動轉換為採 Base64URL 編碼的值或加以轉換。
這些 API 適用於 Chrome 129。
建立密碼金鑰前,請擷取經過編碼的 JSON 檔案
從伺服器擷取 PublicKeyCredentialCreationOptions
物件,並使用該物件解碼
PublicKeyCredential.parseCreationOptionsFromJSON()
。
export async function registerCredential() {
// Fetch encoded `PublicKeyCredentialCreationOptions`
// and JSON decode it.
const options = await fetch('/auth/registerRequest').json();
// Decode `PublicKeyCredentialCreationOptions` JSON object
const decodedOptions = PublicKeyCredential.parseCreationOptionsFromJSON(options);
// Invoke the WebAuthn create() function.
const cred = await navigator.credentials.create({
publicKey: decodedOptions,
});
...
建立密碼金鑰後,請使用 toJSON()
為產生的憑證編碼,以便讓系統
這可以表示它可以傳送至伺服器
...
const cred = await navigator.credentials.create({
publicKey: options,
});
// Encode the credential to JSON and stringify
const credential = JSON.stringify(cred.toJSON());
// Send the encoded credential to the server
await fetch('/auth/registerResponse', credential);
...
使用密碼金鑰進行驗證前,請先擷取經過 JSON 編碼的 JSON
伺服器中的 PublicKeyRequestCreationOptions
,並使用該用戶端對其解碼
PublicKeyCredential.parseRequestOptionsFromJSON()
。
export async function authenticate() {
// Fetch encoded `PublicKeyCredentialRequestOptions`
// and JSON decode it.
const options = await fetch('/auth/signinRequest').json();
// Decode `PublicKeyCredentialRequestOptions` JSON object
const decodedOptions = PublicKeyCredential.parseRequestOptionsFromJSON(options);
// Invoke the WebAuthn get() function.
const cred = await navigator.credentials.get({
publicKey: options
});
...
使用密碼金鑰進行驗證後,請使用以下程式碼為產生的憑證編碼:
toJSON()
方法,以便傳送至伺服器。
...
const cred = await navigator.credentials.get({
publicKey: options
});
// Encode the credential to JSON and stringify
const credential = JSON.stringify(cred.toJSON());
// Send the encoded credential to the server
await fetch(`/auth/signinResponse`, credential);
...
瞭解詳情
如要進一步瞭解 WebAuthn 和密碼金鑰,請參閱下列資源: