オンラインショッピングでは、大量の商品を見て 圧倒されがちです レビューや商品数などですどのようにして 特定のニーズを満たす商品を見つけるにはどうすればよいでしょうか。
たとえば、仕事用のバックパックを購入するとします。バックパックは 機能、デザイン、実用性のバランスを取る必要があります。レビューの数によって、 完璧なバッグが見つかればそれを知ることはほぼ不可能です。もし何ができるとしたら AI を使ってノイズを選別し、最適な製品を見つけるにはどうすればよいでしょうか。
参考になるのは、すべてのレビューの概要と、 一般的な長所と短所を確認します。
<ph type="x-smartling-placeholder">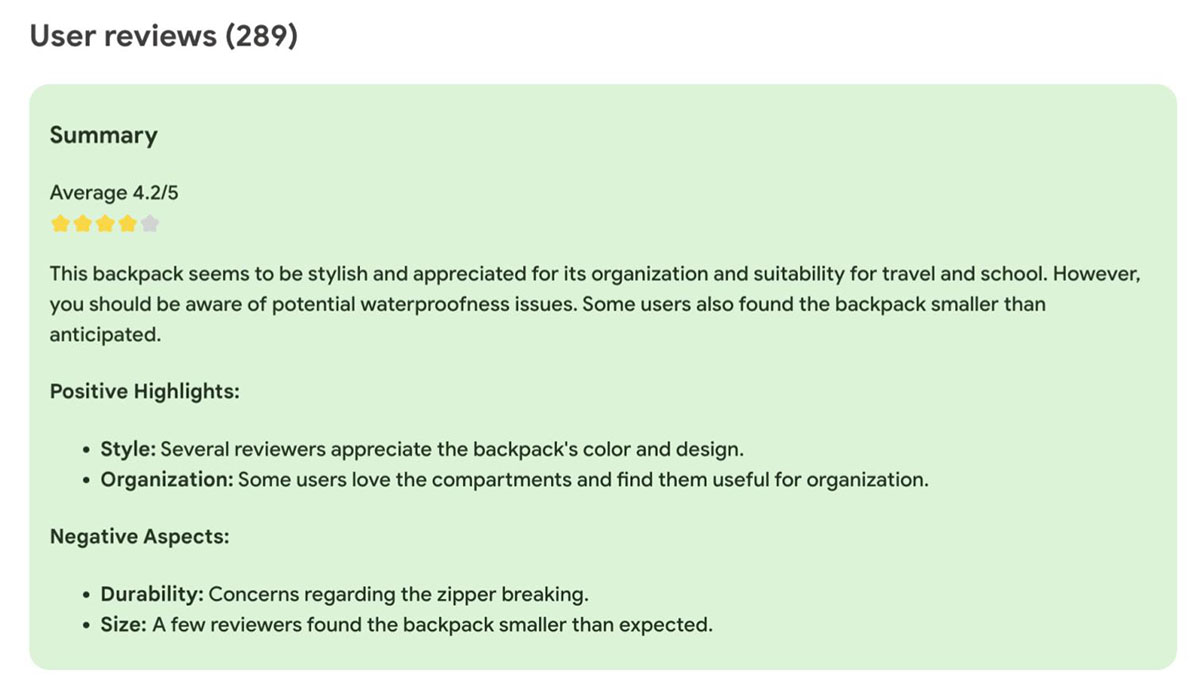
これを構築するために、サーバーサイドの生成 AI を使用しています。推論はサーバー上で行われます。
このドキュメントでは、Google Chat の機能を Node.js を使用した Gemini API を使用して多くのレビューのデータを要約します。重点分野 生成 AI についてお話しします結果の保存方法については ジョブキューを作成できます
実際には、任意の SDK で任意の LLM API を使用できます。ただし、 選択したモデルに合わせて、提案されるプロンプトを適応させる必要がある場合があります。
前提条件
Gemini API のキーを作成します。 環境ファイルで定義します。
Google AI JavaScript SDK をインストールします(npm などを使用)。
npm install @google/generative-ai
クチコミ要約アプリケーションを作成する
- 生成 AI オブジェクトを初期化します。
- クチコミの概要を生成する関数を作成します。
- 生成 AI モデルを選択します。このユースケースでは、Gemini Pro を使用します。使用
ユースケースに固有のモデル(例:
gemini-pro-vision
) マルチモーダル入力用です)。 - プロンプトを追加します。
generateContent
を呼び出して、プロンプトを引数として渡します。- レスポンスを生成して返します。
- 生成 AI モデルを選択します。このユースケースでは、Gemini Pro を使用します。使用
ユースケースに固有のモデル(例:
const { GoogleGenerativeAI } = require("@google/generative-ai");
// Access the API key env
const genAI = new GoogleGenerativeAI(process.env.API_KEY_GEMINI);
async function generateReviewSummary(reviews) {
// Use gemini-pro model for text-only input
const model = genAI.getGenerativeModel({ model: "gemini-pro" });
// Shortened for legibility. See "Write an effective prompt" for
// writing an actual production-ready prompt.
const prompt = `Summarize the following product reviews:\n\n${reviews}`;
const result = await model.generateContent(prompt);
const response = await result.response;
const summary = response.text();
return summary;
}
効果的なプロンプトを作成する
生成 AI を効果的に活用する最善の方法は、完全なプロンプトを作成することです。この例では、ワンショット プロンプトの手法を使用して一貫した出力を得ています。
ワンショット プロンプトは、Gemini がモデル化する出力例で表されています。
const prompt =
`I will give you user reviews for a product. Generate a short summary of the
reviews, with focus on the common positive and negative aspects across all of
the reviews. Use the exact same output format as in the example (list of
positive highlights, list of negative aspects, summary). In the summary,
address the potential buyer with second person ("you", "be aware").
Input (list of reviews):
// ... example
Output (summary of reviews):
// ... example
**Positive highlights**
// ... example
**Negative aspects**
// ... example
**Summary**
// ... example
Input (list of reviews):
${reviews}
Output (summary of all input reviews):`;
このプロンプトの出力例には、すべてのアラートの要約が含まれています。 一般的な長所と短所の一覧も示されています。
## Summary of Reviews:
**Positive highlights:**
* **Style:** Several reviewers appreciate the backpack's color and design.
* **Organization:** Some users love the compartments and find them useful for
organization.
* **Travel & School:** The backpack seems suitable for both travel and school
use, being lightweight and able to hold necessary items.
**Negative aspects:**
* **Durability:** Concerns regarding the zipper breaking and water bottle holder
ripping raise questions about the backpack's overall durability.
* **Size:** A few reviewers found the backpack smaller than expected.
* **Material:** One user felt the material was cheap and expressed concern about
its longevity.
**Summary:**
This backpack seems to be stylish and appreciated for its organization and
suitability for travel and school. However, you should be aware of potential
durability issues with the zippers and water bottle holder. Some users also
found the backpack smaller than anticipated and expressed concerns about the
material's quality.
トークンの上限
多くのレビューでモデルのトークン上限に達する可能性があります。トークンは常に同じとは限りません。 1 語トークンは単語の一部であることも、複数の単語が一緒になったこともあります。対象 たとえば、Gemini Pro は 30,720 トークンの上限がありますつまり、プロンプトには最大 600 個の平均 残りのプロンプトの指示は除く、英語の 30 語のレビュー。
countTokens()
を使用する
トークンの数をチェックし、プロンプトが大きければ入力を減らします。
できます。
const MAX_INPUT_TOKENS = 30720
const { totalTokens } = await model.countTokens(prompt);
if (totalTokens > MAX_INPUT_TOKENS) {
// Shorten the prompt.
}
企業向けの開発
Google Cloud ユーザーの場合、またはエンタープライズ サポートが必要な場合、 Gemini Pro やその他のモデル(Anthropic の Claude モデルなど)にアクセスできます。 Vertex AI。これらを使用して、 Model Garden を使用して、どのモデルを使用するかを決定する ユースケースに最適なプロダクトを選択できます
次のステップ
私たちが構築したアプリケーションは、プロダクト オペレーションの効率が 生成 AI です。質の高いレビューを収集するには、 このシリーズは オンデバイスのウェブ AI により、ユーザーが有益な商品レビューを書くことができます。
このアプローチについて、ぜひご意見をお聞かせください。最も多いユースケースを教えてください できます。Google Chat では フィードバックを送信して早期プレビュー プログラムにご参加いただけます ローカルのプロトタイプで このテクノロジーをテストしました
皆様のご協力は、AI を強力かつ実用的なツールにするために役立っています。 できます。