Support WebGPU on Android
The Chrome team is excited to announce that WebGPU is now enabled by default in Chrome 121 on devices running Android 12 and greater powered by Qualcomm and ARM GPUs.
Support will gradually expand to encompass a wider range of Android devices, including those running on Android 11 in a near future. This expansion will be dependent on further testing and optimization to ensure a seamless experience across a broader range of hardware configurations. See issue chromium:1497815.
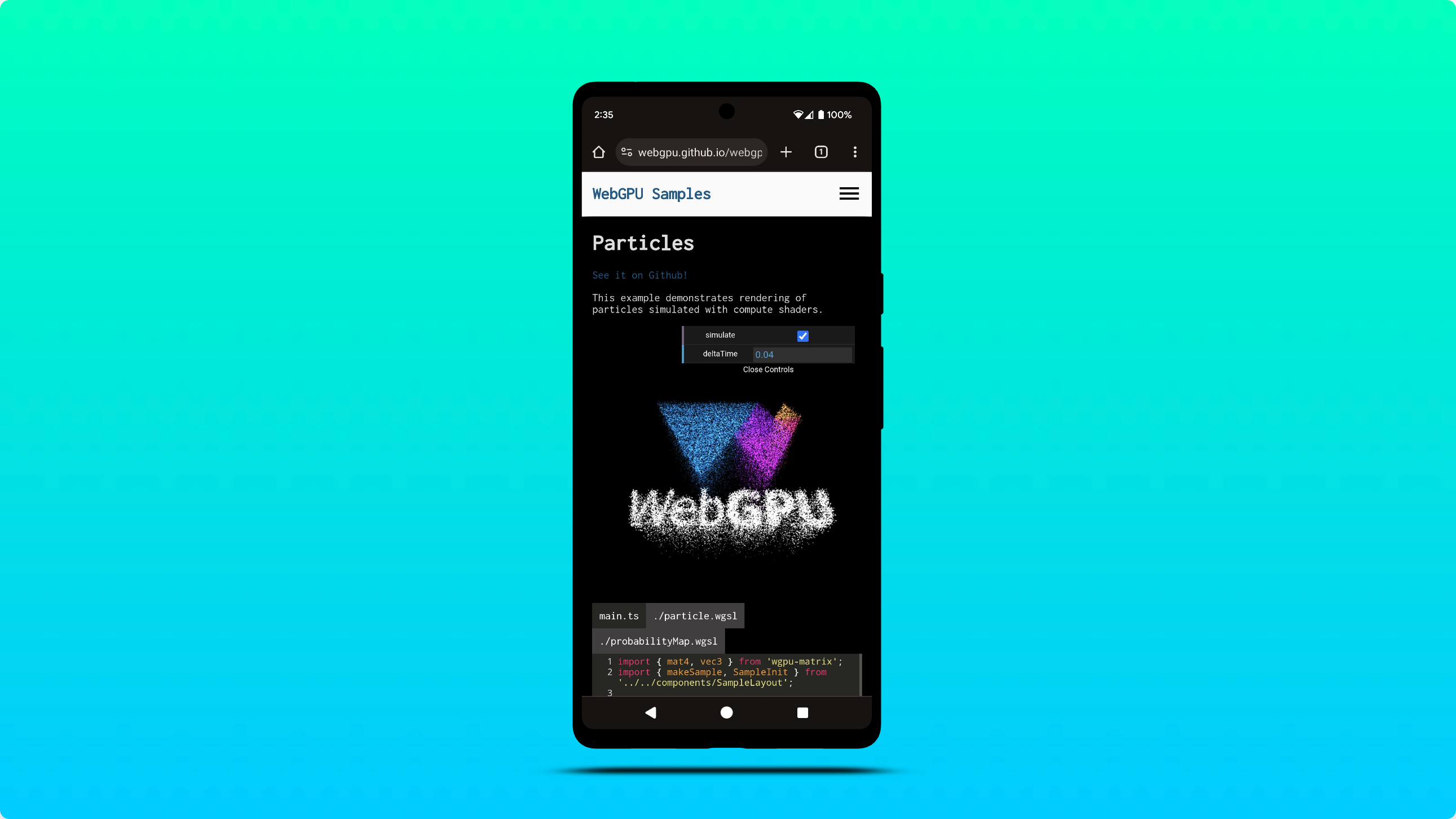
Use DXC instead of FXC for shader compilation on Windows
Chrome now uses the power of DXC (DirectX Compiler) to compile shaders on Windows D3D12 machines equipped with SM6+ graphics hardware. Previously, WebGPU relied on FXC (FX Compiler) for shader compilation on Windows. While functional, FXC lacked the feature set and performance optimizations present in DXC.
Initial testing shows a 20% average increase in compute shader compilation speed when using DXC compared to FXC.
Timestamp queries in compute and render passes
Timestamp queries allow WebGPU applications to measure precisely (down to the nanosecond) how much time their GPU commands take to execute compute and render passes. They are heavily used to gain insights into the performance and behavior of GPU workloads.
When the "timestamp-query"
feature is available in a GPUAdapter
, you can now do the following things:
- Request a
GPUDevice
with the"timestamp-query"
feature. - Create a
GPUQuerySet
of type"timestamp"
. - Use
GPUComputePassDescriptor.timestampWrites
andGPURenderPassDescriptor.timestampWrites
to define where to write timestamp values inGPUQuerySet
. - Resolve timestamp values into a
GPUBuffer
withresolveQuerySet()
. - Read timestamp values back by copying the results from the
GPUBuffer
to the CPU. - Decode timestamp values as a
BigInt64Array
.
See the following example and issue dawn:1800.
const adapter = await navigator.gpu.requestAdapter();
if (!adapter.features.has("timestamp-query")) {
throw new Error("Timestamp query feature is not available");
}
// Explicitly request timestamp query feature.
const device = await adapter.requestDevice({
requiredFeatures: ["timestamp-query"],
});
const commandEncoder = device.createCommandEncoder();
// Create a GPUQuerySet which holds 2 timestamp query results: one for the
// beginning and one for the end of compute pass execution.
const querySet = device.createQuerySet({ type: "timestamp", count: 2 });
const timestampWrites = {
querySet,
beginningOfPassWriteIndex: 0, // Write timestamp in index 0 when pass begins.
endOfPassWriteIndex: 1, // Write timestamp in index 1 when pass ends.
};
const passEncoder = commandEncoder.beginComputePass({ timestampWrites });
// TODO: Set pipeline, bind group, and dispatch work to be performed.
passEncoder.end();
// Resolve timestamps in nanoseconds as a 64-bit unsigned integer into a GPUBuffer.
const size = 2 * BigInt64Array.BYTES_PER_ELEMENT;
const resolveBuffer = device.createBuffer({
size,
usage: GPUBufferUsage.QUERY_RESOLVE | GPUBufferUsage.COPY_SRC,
});
commandEncoder.resolveQuerySet(querySet, 0, 2, resolveBuffer, 0);
// Read GPUBuffer memory.
const resultBuffer = device.createBuffer({
size,
usage: GPUBufferUsage.COPY_DST | GPUBufferUsage.MAP_READ,
});
commandEncoder.copyBufferToBuffer(resolveBuffer, 0, resultBuffer, 0, size);
// Submit commands to the GPU.
device.queue.submit([commandEncoder.finish()]);
// Log compute pass duration in nanoseconds.
await resultBuffer.mapAsync(GPUMapMode.READ);
const times = new BigInt64Array(resultBuffer.getMappedRange());
console.log(`Compute pass duration: ${Number(times[1] - times[0])}ns`);
resultBuffer.unmap();
Due to timing attack concerns, timestamp queries are quantized with a resolution of 100 microseconds, which provides a good compromise between precision and security. In Chrome browser, you can disable timestamp quantization by enabling the "WebGPU Developer Features" flag at chrome://flags/#enable-webgpu-developer-features
during the development of your app. See Timestamp queries quantization to learn more.
As GPUs may reset the timestamp counter occasionally, which can result in unexpected values such as negative deltas between timestamps, I recommend you check out the git diff changes that adds timestamp query support to the following Compute Boids sample.
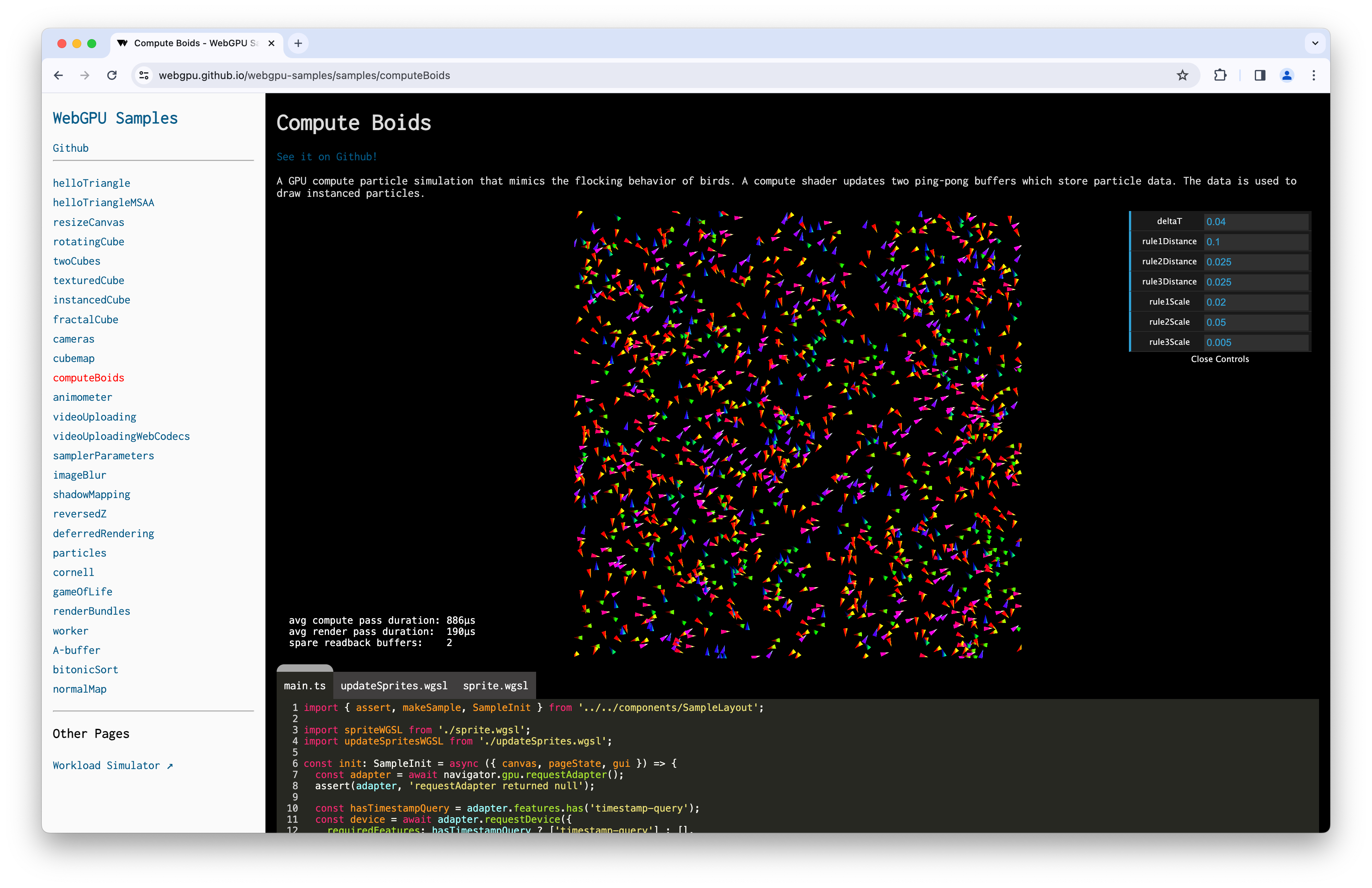
Default entry points to shader modules
To improve the developer experience, you can now omit the entryPoint
of your shader module when creating a compute or render pipeline. If no unique entry point for the shader stage is found in the shader code, a GPUValidationError will be triggered. See the following example and issue dawn:2254.
const code = `
@vertex fn vertexMain(@builtin(vertex_index) i : u32) ->
@builtin(position) vec4f {
const pos = array(vec2f(0, 1), vec2f(-1, -1), vec2f(1, -1));
return vec4f(pos[i], 0, 1);
}
@fragment fn fragmentMain() -> @location(0) vec4f {
return vec4f(1, 0, 0, 1);
}`;
const module = myDevice.createShaderModule({ code });
const format = navigator.gpu.getPreferredCanvasFormat();
const pipeline = await myDevice.createRenderPipelineAsync({
layout: "auto",
vertex: { module, entryPoint: "vertexMain" },
fragment: { module, entryPoint: "fragmentMain", targets: [{ format }] },
vertex: { module },
fragment: { module, targets: [{ format }] },
});
Support display-p3 as GPUExternalTexture color space
You can now set "display-p3"
destination color space when importing a GPUExternalTexture from HDR videos with importExternalTexture()
. Check out how WebGPU handles color spaces. See the following example and issue chromium:1330250.
// Create texture from HDR video.
const video = document.querySelector("video");
const texture = myDevice.importExternalTexture({
source: video,
colorSpace: "display-p3",
});
Memory heaps info
To help you anticipate memory limitations when allocating large amounts during the development of your app, requestAdapterInfo()
now exposes memoryHeaps
information such as the size and type of memory heaps available on the adapter. This experimental feature is accessible only when the "WebGPU Developer Features" flag at chrome://flags/#enable-webgpu-developer-features
is enabled. See the following example and issue dawn:2249.
const adapter = await navigator.gpu.requestAdapter();
const adapterInfo = await adapter.requestAdapterInfo();
for (const { size, properties } of adapterInfo.memoryHeaps) {
console.log(size); // memory heap size in bytes
if (properties & GPUHeapProperty.DEVICE_LOCAL) { /* ... */ }
if (properties & GPUHeapProperty.HOST_VISIBLE) { /* ... */ }
if (properties & GPUHeapProperty.HOST_COHERENT) { /* ... */ }
if (properties & GPUHeapProperty.HOST_UNCACHED) { /* ... */ }
if (properties & GPUHeapProperty.HOST_CACHED) { /* ... */ }
}
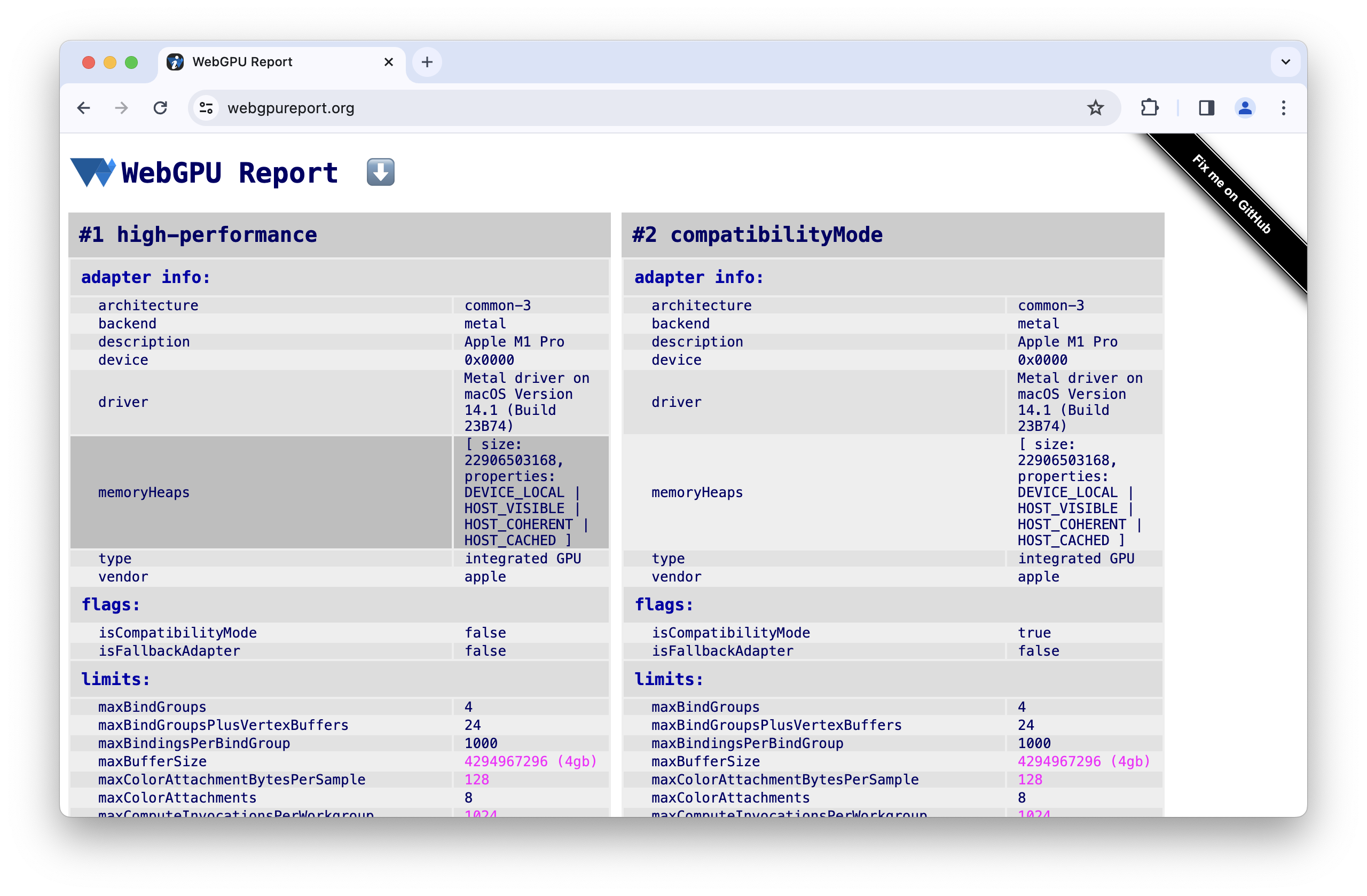
Dawn updates
The HasWGSLLanguageFeature
and EnumerateWGSLLanguageFeatures
methods on wgpu::Instance
have been added to handle WGSL language features. See issue dawn:2260.
The non-standard wgpu::Feature::BufferMapExtendedUsages
feature lets you create a GPU buffer with wgpu::BufferUsage::MapRead
or wgpu::BufferUsage::MapWrite
and any other wgpu::BufferUsage
. See the following example and issue dawn:2204.
wgpu::BufferDescriptor descriptor = {
.size = 128,
.usage = wgpu::BufferUsage::MapWrite | wgpu::BufferUsage::Uniform
};
wgpu::Buffer uniformBuffer = device.CreateBuffer(&descriptor);
uniformBuffer.MapAsync(wgpu::MapMode::Write, 0, 128,
[](WGPUBufferMapAsyncStatus status, void* userdata)
{
wgpu::Buffer* buffer = static_cast<wgpu::Buffer*>(userdata);
memcpy(buffer->GetMappedRange(), data, sizeof(data));
},
&uniformBuffer);
The following features have been documented: ANGLE Texture Sharing, D3D11 multithread protected, Implicit Device Synchronization, Norm16 texture formats, Timestamp Query Inside Passes, Pixel Local Storage, Shader Features, and Multi Planar Formats.
The Chrome team has created an official GitHub repository for Dawn.
This covers only some of the key highlights. Check out the exhaustive list of commits.
What's New in WebGPU
A list of everything that has been covered in the What's New in WebGPU series.
Chrome 131
- Clip distances in WGSL
- GPUCanvasContext getConfiguration()
- Point and line primitives must not have depth bias
- Inclusive scan built-in functions for subgroups
- Experimental support for multi-draw indirect
- Shader module compilation option strict math
- Remove GPUAdapter requestAdapterInfo()
- Dawn updates
Chrome 130
- Dual source blending
- Shader compilation time improvements on Metal
- Deprecation of GPUAdapter requestAdapterInfo()
- Dawn updates
Chrome 129
Chrome 128
- Experimenting with subgroups
- Deprecate setting depth bias for lines and points
- Hide uncaptured error DevTools warning if preventDefault
- WGSL interpolate sampling first and either
- Dawn updates
Chrome 127
- Experimental support for OpenGL ES on Android
- GPUAdapter info attribute
- WebAssembly interop improvements
- Improved command encoder errors
- Dawn updates
Chrome 126
- Increase maxTextureArrayLayers limit
- Buffer upload optimization for Vulkan backend
- Shader compilation time improvements
- Submitted command buffers must be unique
- Dawn updates
Chrome 125
Chrome 124
- Read-only and read-write storage textures
- Service workers and shared workers support
- New adapter information attributes
- Bug fixes
- Dawn updates
Chrome 123
- DP4a built-in functions support in WGSL
- Unrestricted pointer parameters in WGSL
- Syntax sugar for dereferencing composites in WGSL
- Separate read-only state for stencil and depth aspects
- Dawn updates
Chrome 122
- Expand reach with compatibility mode (feature in development)
- Increase maxVertexAttributes limit
- Dawn updates
Chrome 121
- Support WebGPU on Android
- Use DXC instead of FXC for shader compilation on Windows
- Timestamp queries in compute and render passes
- Default entry points to shader modules
- Support display-p3 as GPUExternalTexture color space
- Memory heaps info
- Dawn updates
Chrome 120
- Support for 16-bit floating-point values in WGSL
- Push the limits
- Changes to depth-stencil state
- Adapter information updates
- Timestamp queries quantization
- Spring-cleaning features
Chrome 119
- Filterable 32-bit float textures
- unorm10-10-10-2 vertex format
- rgb10a2uint texture format
- Dawn updates
Chrome 118
- HTMLImageElement and ImageData support in
copyExternalImageToTexture()
- Experimental support for read-write and read-only storage texture
- Dawn updates
Chrome 117
- Unset vertex buffer
- Unset bind group
- Silence errors from async pipeline creation when device is lost
- SPIR-V shader module creation updates
- Improving developer experience
- Caching pipelines with automatically generated layout
- Dawn updates
Chrome 116
- WebCodecs integration
- Lost device returned by GPUAdapter
requestDevice()
- Keep video playback smooth if
importExternalTexture()
is called - Spec conformance
- Improving developer experience
- Dawn updates
Chrome 115
- Supported WGSL language extensions
- Experimental support for Direct3D 11
- Get discrete GPU by default on AC power
- Improving developer experience
- Dawn updates
Chrome 114
- Optimize JavaScript
- getCurrentTexture() on unconfigured canvas throws InvalidStateError
- WGSL updates
- Dawn updates