Published: March 26, 2025
Allow creating pipeline layout with null bind group layout
Previously, creating an empty bind group layout required adding a bind group with zero bindings, which was inconvenient. This is no longer necessary as null bind group layouts are now allowed and ignored when creating a pipeline layout. This should make development easier.
For example, you might want to create a pipeline that uses only bind group layouts 0 and 2. You could assign bind group layout 1 to fragment data and bind group layout 2 to vertex data, and then render without a fragment shader. See issue 377836524.
const bgl0 = myDevice.createBindGroupLayout({ entries: myGlobalEntries });
const bgl1 = myDevice.createBindGroupLayout({ entries: myFragmentEntries });
const bgl2 = myDevice.createBindGroupLayout({ entries: myVertexEntries });
// Create a pipeline layout that will be used to render without a fragment shader.
const myPipelineLayout = myDevice.createPipelineLayout({
bindGroupLayouts: [bgl0, null, bgl2],
});
Allow viewports to extend past the render targets bounds
The requirements for viewport validation have been relaxed to allow viewports to go beyond the render target boundaries. This is especially useful for drawing 2D elements such as UI that may extend outside the current viewport. See issue 390162929.
const passEncoder = myCommandEncoder.beginRenderPass({
colorAttachments: [
{
view: myColorTexture.createView(),
loadOp: "clear",
storeOp: "store",
},
],
});
// Set a viewport that extends past the render target's bounds by 8 pixels
// in all directions.
passEncoder.setViewport(
/*x=*/ -8,
/*y=*/ -8,
/*width=*/ myColorTexture.width + 16,
/*height=*/ myColorTexture.height + 16,
/*minDepth=*/ 0,
/*maxDepth=*/ 1,
);
// Draw geometry and complete the render pass as usual.
Easier access to the experimental compatibility mode on Android
The chrome://flags/#enable-unsafe-webgpu
flag alone now enables all capabilities required for the experimental WebGPU compatibility mode on Android. With that, you can request a GPUAdapter in compatibility mode with the featureLevel: "compatibility"
option and even get access to the OpenGL ES backend on devices lacking support for Vulkan. See the following example and issue dawn:389876644.
// Request a GPUAdapter in compatibility mode.
const adapter = await navigator.gpu.requestAdapter({ featureLevel: "compatibility" });
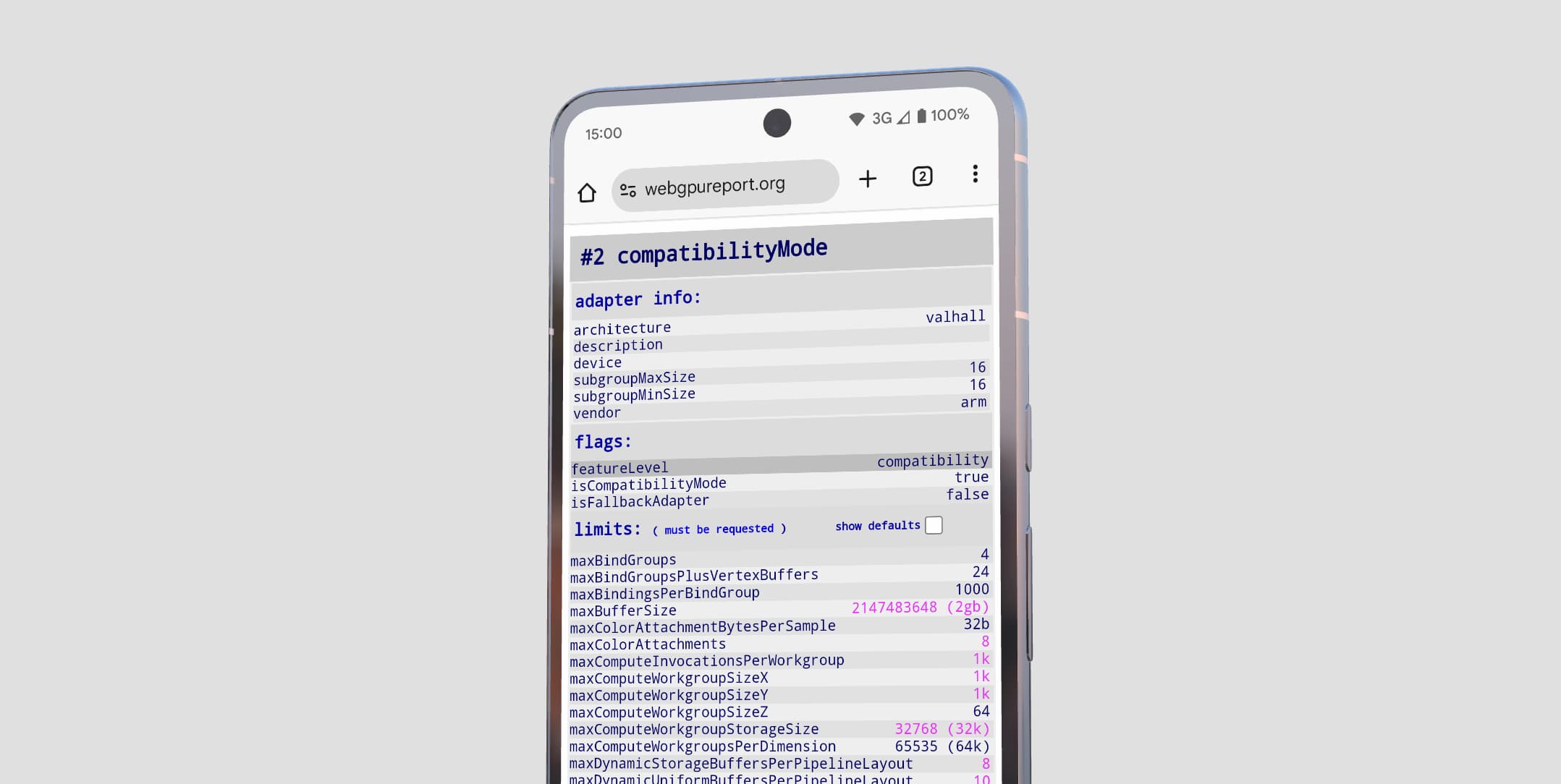
Remove maxInterStageShaderComponents limit
As previously announced, the maxInterStageShaderComponents limit is removed due to a combination of factors:
- Redundancy with
maxInterStageShaderVariables
: This limit already serves a similar purpose, controlling the amount of data passed between shader stages. - Minor discrepancies: While there are slight differences in how the two limits are calculated, these differences are minor and can be effectively managed within the
maxInterStageShaderVariables
limit. - Simplification: Removing
maxInterStageShaderComponents
streamlines the shader interface and reduces complexity for developers. Instead of managing two separate limits with subtle differences, they can focus on the more appropriately named and comprehensivemaxInterStageShaderVariables
.
See intent to remove and issue 364338810.
Dawn updates
It's no longer possible to use a filtering sampler to sample a depth texture. As a reminder, a depth texture can only be used with a non filtering or a comparison sampler. See issue 379788112.
The WGPURequiredLimits
and WGPUSupportedLimits
structures have been flattened into WGPULimits
. See issue 374263404.
The following structs have been renamed. See issue 42240793.
WGPUImageCopyBuffer
is nowWGPUTexelCopyBufferInfo
WGPUImageCopyTexture
is nowWGPUTexelCopyTextureInfo
WGPUTextureDataLayout
is nowWGPUTexelCopyBufferLayout
The subgroupMinSize
and subgroupMaxSize
members have been added to the WGPUAdapterInfo
struct. See webgpu-headers PR.
Tracing Dawn API usage in Metal is now possible when running your program with the DAWN_TRACE_FILE_BASE
environment variable which saves a .gputrace file that can be loaded later into XCode's Metal Debugger. See the Debugging Dawn documentation.
This covers only some of the key highlights. Check out the exhaustive list of commits.
What's New in WebGPU
A list of everything that has been covered in the What's New in WebGPU series.
Chrome 135
- Allow creating pipeline layout with null bind group layout
- Allow viewports to extend past the render targets bounds
- Easier access to the experimental compatibility mode on Android
- Remove maxInterStageShaderComponents limit
- Dawn updates
Chrome 134
- Improve machine-learning workloads with subgroups
- Remove float filterable texture types support as blendable
- Dawn updates
Chrome 133
- Additional unorm8x4-bgra and 1-component vertex formats
- Allow unknown limits to be requested with undefined value
- WGSL alignment rules changes
- WGSL performance gains with discard
- Use VideoFrame displaySize for external textures
- Handle images with non-default orientations using copyExternalImageToTexture
- Improving developer experience
- Enable compatibility mode with featureLevel
- Experimental subgroup features cleanup
- Deprecate maxInterStageShaderComponents limit
- Dawn updates
Chrome 132
- Texture view usage
- 32-bit float textures blending
- GPUDevice adapterInfo attribute
- Configuring canvas context with invalid format throw JavaScript error
- Filtering sampler restrictions on textures
- Extended subgroups experimentation
- Improving developer experience
- Experimental support for 16-bit normalized texture formats
- Dawn updates
Chrome 131
- Clip distances in WGSL
- GPUCanvasContext getConfiguration()
- Point and line primitives must not have depth bias
- Inclusive scan built-in functions for subgroups
- Experimental support for multi-draw indirect
- Shader module compilation option strict math
- Remove GPUAdapter requestAdapterInfo()
- Dawn updates
Chrome 130
- Dual source blending
- Shader compilation time improvements on Metal
- Deprecation of GPUAdapter requestAdapterInfo()
- Dawn updates
Chrome 129
Chrome 128
- Experimenting with subgroups
- Deprecate setting depth bias for lines and points
- Hide uncaptured error DevTools warning if preventDefault
- WGSL interpolate sampling first and either
- Dawn updates
Chrome 127
- Experimental support for OpenGL ES on Android
- GPUAdapter info attribute
- WebAssembly interop improvements
- Improved command encoder errors
- Dawn updates
Chrome 126
- Increase maxTextureArrayLayers limit
- Buffer upload optimization for Vulkan backend
- Shader compilation time improvements
- Submitted command buffers must be unique
- Dawn updates
Chrome 125
Chrome 124
- Read-only and read-write storage textures
- Service workers and shared workers support
- New adapter information attributes
- Bug fixes
- Dawn updates
Chrome 123
- DP4a built-in functions support in WGSL
- Unrestricted pointer parameters in WGSL
- Syntax sugar for dereferencing composites in WGSL
- Separate read-only state for stencil and depth aspects
- Dawn updates
Chrome 122
- Expand reach with compatibility mode (feature in development)
- Increase maxVertexAttributes limit
- Dawn updates
Chrome 121
- Support WebGPU on Android
- Use DXC instead of FXC for shader compilation on Windows
- Timestamp queries in compute and render passes
- Default entry points to shader modules
- Support display-p3 as GPUExternalTexture color space
- Memory heaps info
- Dawn updates
Chrome 120
- Support for 16-bit floating-point values in WGSL
- Push the limits
- Changes to depth-stencil state
- Adapter information updates
- Timestamp queries quantization
- Spring-cleaning features
Chrome 119
- Filterable 32-bit float textures
- unorm10-10-10-2 vertex format
- rgb10a2uint texture format
- Dawn updates
Chrome 118
- HTMLImageElement and ImageData support in
copyExternalImageToTexture()
- Experimental support for read-write and read-only storage texture
- Dawn updates
Chrome 117
- Unset vertex buffer
- Unset bind group
- Silence errors from async pipeline creation when device is lost
- SPIR-V shader module creation updates
- Improving developer experience
- Caching pipelines with automatically generated layout
- Dawn updates
Chrome 116
- WebCodecs integration
- Lost device returned by GPUAdapter
requestDevice()
- Keep video playback smooth if
importExternalTexture()
is called - Spec conformance
- Improving developer experience
- Dawn updates
Chrome 115
- Supported WGSL language extensions
- Experimental support for Direct3D 11
- Get discrete GPU by default on AC power
- Improving developer experience
- Dawn updates
Chrome 114
- Optimize JavaScript
- getCurrentTexture() on unconfigured canvas throws InvalidStateError
- WGSL updates
- Dawn updates