Develop
After reading the Get Started section, use this guide as an outline of extension components, their capabilities in Manifest V3 and how to combine them. First familiarize yourself with what extensions are capable of: Then learn how to combine these features using the extensions core concepts section.
Design the user interface
Most extensions need some kind of user interaction to work. The extensions platform provides a variety of ways to add interactions to your extension. These methods include popups triggered from the Chrome toolbar, side panels, context menus, and more.
Side panel
Use the
chrome.sidePanel
API to host content in the browser's side panel alongside the main content of a web page.
Action
Control the display of an extension's icon in the toolbar.
Menus
Add items to Google Chrome's context menu.
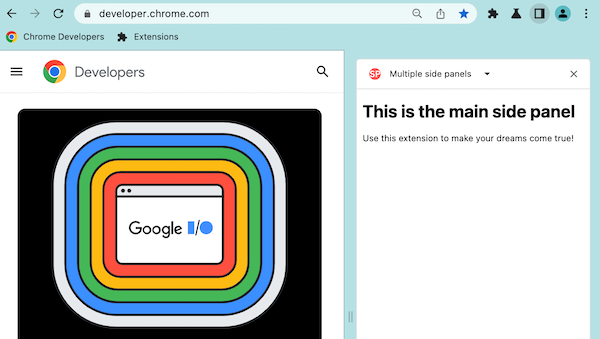
Control the browser
Chrome's extension APIs make it possible to change the way your browser works.
Override Chrome pages and settings
Settings overrides are a way for extensions to override selected Chrome settings. Additionally, extensions can use HTML override pages to replace a page Google Chrome normally provides. An extension can override the bookmark manager, the history tab, or the new tab.
Extending DevTools
DevTools extensions add functionality to Chrome DevTools by accessing DevTools-specific extension APIs through a DevTools page added to the extension. You can also use the
chrome.debugger
API to invoke Chrome's remote debugging protocol. Attach to one or more tabs to instrument network interaction, debug JavaScript, mutate the DOM, and more.
Display notifications
The
chrome.notifications
API lets you create notifications using templates and show these notifications to users in the user's system tray.
Manage history
Use the
chrome.history
API to interact with the browser's record of visited pages, and the chrome.browsingData
API to manage other browsing data. Use chrome.topSites
to access the most visited sites.
Control tabs and windows
Use APIs such as
chrome.tabs
, chrome.tabGroups
and chrome.windows
to create, modify, and arrange the user's browser.
Add keyboard shortcuts
Use the
chrome.commands
API to add keyboard shortcuts that trigger actions in your extension. For example, you can add a shortcut to open the browser action or send a command to the extension.
Authenticate users
Use the
chrome.identity
API to get OAuth 2.0 access tokens.
Manage extensions
The
chrome.management
API provides ways to manage the list of extensions that are installed and running. It is particularly useful for extensions that override the built-in New Tab page.
Provide suggestions
The
chrome.omnibox
API allows you to register a keyword with Google Chrome's omnibox (address bar).
Update Chrome settings
Use the
chrome.privacy
API to control usage of features in Chrome that can affect a user's privacy. Also see the chrome.proxy
API to manage Chrome's proxy settings.
Manage downloads
Use the
chrome.downloads
API to programmatically initiate, monitor, manipulate, and search for downloads.
Use bookmarks and the reading list
Use the
chrome.bookmarks
API and the chrome.readingList
API to create, organize, and otherwise manipulate these lists.
Control the web
Dynamically change the content and behavior of web pages. You can control and modify the web by injecting scripts, intercepting network requests, and using web APIs to interact with web pages.
Inject JavaScript and CSS
Content scripts are files that run in the context of web pages. They use the standard Document Object Model (DOM), to read details of web pages the browser visits, make changes to them, and pass information to their parent extension.
Access the active tab
The
"activeTab"
permission gives an extension temporary access to the currently active tab when the user invokes the extension, for example by clicking its action. Access to the tab lasts while the user is on that page, and is revoked when the user navigates away or closes the tab.
Control web requests
Use the
chrome.declarativeNetRequest
, chrome.webRequest
and chrome.webNavigation
APIs to observe, block, and modify network requests.
Audio recording and screen capture
Learn about different approaches for recording audio and video from a tab, window, or screen using web platform APIs such as
chrome.tabCapture
or getDisplayMedia()
.
Modify website settings
Use the
chrome.contentSettings
API to control whether websites can use features such as cookies, JavaScript, and plugins. More generally speaking, content settings allow you to customize Chrome's behavior on a per-site basis instead of globally.
Core concepts
Using web platform and extension APIs you can build more complex features by combining different UI components and extension platform features.
Service workers
An extension service worker (service-worker.js) is an event-based script that the browser runs in the background. It is often used to process data, coordinate tasks in different parts of an extension, and as an extension's event manager.
Permissions
Understand permissions: how they work and when to avoid asking for them when they're not needed.
Messaging
Many times content scripts, or other extension pages, need to send or receive information from the extension service worker. In these cases, either side can listen for messages sent from the other end, and respond on the same channel.
Native messaging
Enable your extensions to exchange messages with native applications.
Avoid remotely hosted code
In Manifest V3 extensions need to bundle all code they are using inside the extension itself. There are different strategies for doing this.
Storage
Chrome Extensions have a specialized Storage API, available to all extension components. It includes four separate storage areas for specific use cases and an event listener that tracks whenever data is updated.
Offscreen documents
Service workers don't have DOM access. The Offscreen API allows the extension to use DOM APIs in a hidden document without interrupting the user experience by opening new windows or tabs.
Cross origin isolation
Cross-origin isolation enables a web page to use powerful features such as
SharedArrayBuffer
. An extension can opt into cross-origin isolation by specifying the appropriate values for the "cross_origin_embedder_policy"
and "cross_origin_opener_policy"
manifest keys.