このガイドでは、タブ、ウィンドウ、またはデバイスから音声と動画を録画するさまざまな方法について説明します
chrome.tabCapture
や
getDisplayMedia()
。
画面の録画
画面の録画の場合は、getDisplayMedia()
を呼び出します。これにより、ダイアログ ボックスがトリガーされます。
表示されます。これにより、ユーザーは使用するタブ、ウィンドウ、画面を選択できるようになります。
録画が行われていることが明確に示されます。
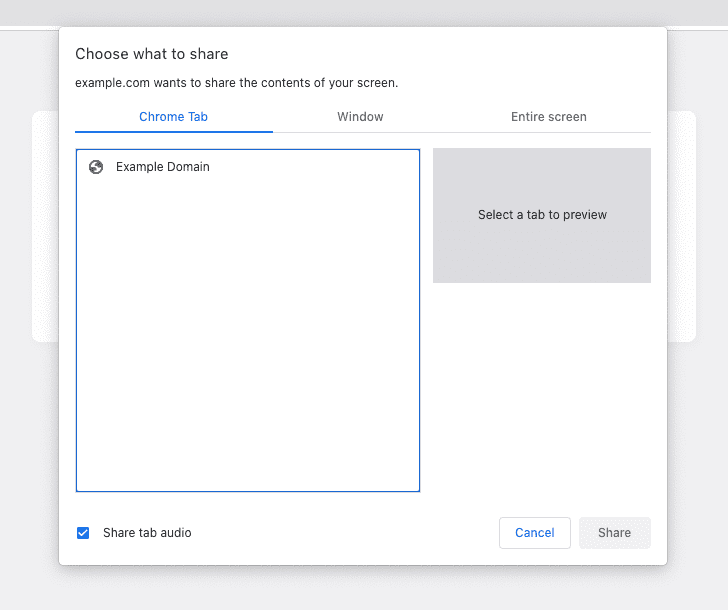
次の例では、音声と動画の両方を記録するアクセス権をリクエストしています。
const stream = await navigator.mediaDevices.getDisplayMedia({ audio: true, video: true });
コンテンツ スクリプト内で呼び出された場合、ユーザーが新しいコンテンツに移動すると、録画は自動的に終了します。
できます。バックグラウンドやナビゲーションをまたいで録画するには、
オフスクリーン ドキュメントに DISPLAY_MEDIA
の理由が表示されます。
ユーザー操作に基づくタブキャプチャ
getDisplayMedia()
を呼び出すと、ブラウザには、
選択することもできます。ただし、ユーザーがページをクリックし、
操作ボタンをクリックして、特定のタブで拡張機能を呼び出し、
このメッセージは表示されず、すぐにタブのキャプチャが開始されます。
バックグラウンドで音声と動画を録画
Chrome 116 以降では、Service Worker で chrome.tabCapture
API を呼び出すことができます。
を使用してストリーム ID を取得できます。これを画面外のドキュメントに渡し、
録画を開始します。
Service Worker で以下を行います。
chrome.action.onClicked.addListener(async (tab) => {
const existingContexts = await chrome.runtime.getContexts({});
const offscreenDocument = existingContexts.find(
(c) => c.contextType === 'OFFSCREEN_DOCUMENT'
);
// If an offscreen document is not already open, create one.
if (!offscreenDocument) {
// Create an offscreen document.
await chrome.offscreen.createDocument({
url: 'offscreen.html',
reasons: ['USER_MEDIA'],
justification: 'Recording from chrome.tabCapture API',
});
}
// Get a MediaStream for the active tab.
const streamId = await chrome.tabCapture.getMediaStreamId({
targetTabId: tab.id
});
// Send the stream ID to the offscreen document to start recording.
chrome.runtime.sendMessage({
type: 'start-recording',
target: 'offscreen',
data: streamId
});
});
次に、画面外のドキュメントで次の操作を行います。
chrome.runtime.onMessage.addListener(async (message) => {
if (message.target !== 'offscreen') return;
if (message.type === 'start-recording') {
const media = await navigator.mediaDevices.getUserMedia({
audio: {
mandatory: {
chromeMediaSource: "tab",
chromeMediaSourceId: message.data,
},
},
video: {
mandatory: {
chromeMediaSource: "tab",
chromeMediaSourceId: message.data,
},
},
});
// Continue to play the captured audio to the user.
const output = new AudioContext();
const source = output.createMediaStreamSource(media);
source.connect(output.destination);
// TODO: Do something to recording the MediaStream.
}
});
詳細な例については、タブキャプチャ - レコーダーのサンプルをご覧ください。
新しいタブで音声と動画を録画する
Chrome 116 より前のバージョンでは、chrome.tabCapture
API を
または、その API によって作成されたストリーム ID を画面外のドキュメントで使用することもできます。上記の両方
要件です
代わりに、拡張機能のページを新しいタブまたはウィンドウで開き、ストリームを直接取得できます。セット
targetTabId
プロパティ: 正しいタブをキャプチャします。
まず、拡張機能ページを開きます(ポップアップや Service Worker などで開きます)。
chrome.windows.create({ url: chrome.runtime.getURL("recorder.html") });
次に、拡張機能ページで次の操作を行います。
chrome.tabCapture.getMediaStreamId({ targetTabId: tabId }, async (id) => {
const media = await navigator.mediaDevices.getUserMedia({
audio: {
mandatory: {
chromeMediaSource: "tab",
chromeMediaSourceId: id,
},
},
video: {
mandatory: {
chromeMediaSource: "tab",
chromeMediaSourceId: id,
},
},
});
// Continue to play the captured audio to the user.
const output = new AudioContext();
const source = output.createMediaStreamSource(media);
source.connect(output.destination);
});
または、画面の録画の手法を使用すると、 オフスクリーン ドキュメントを使用してバックグラウンドで記録しますが、ユーザーにはタブ選択ダイアログが表示されます。 クリックします。
ポップアップで音声を録音する
音声の録音のみが必要な場合は、拡張機能のポップアップで chrome.tabCapture.capture:ポップアップが閉じると、録画が停止します。
chrome.tabCapture.capture({ audio: true }, (stream) => {
// Continue to play the captured audio to the user.
const output = new AudioContext();
const source = output.createMediaStreamSource(stream);
source.connect(output.destination);
// TODO: Do something with the stream (e.g record it)
});
ナビゲーション間で録音を保持する必要がある場合は、ここで説明する方法の使用を検討してください。 (前のセクションをご覧ください)
その他の考慮事項
ストリームを録画する方法について詳しくは、MediaRecorder API をご覧ください。